Introduction
In this project, we will create a simple clock animation using OpenGL and GLUT (Graphics Library Utility Toolkit). This animation will display a clock with moving clock hands to represent the current time. The clock will update in real-time, simulating the movement of the hour, minute, and second hands. We will start by setting up the project files and then proceed with the necessary code.
👀 Preview
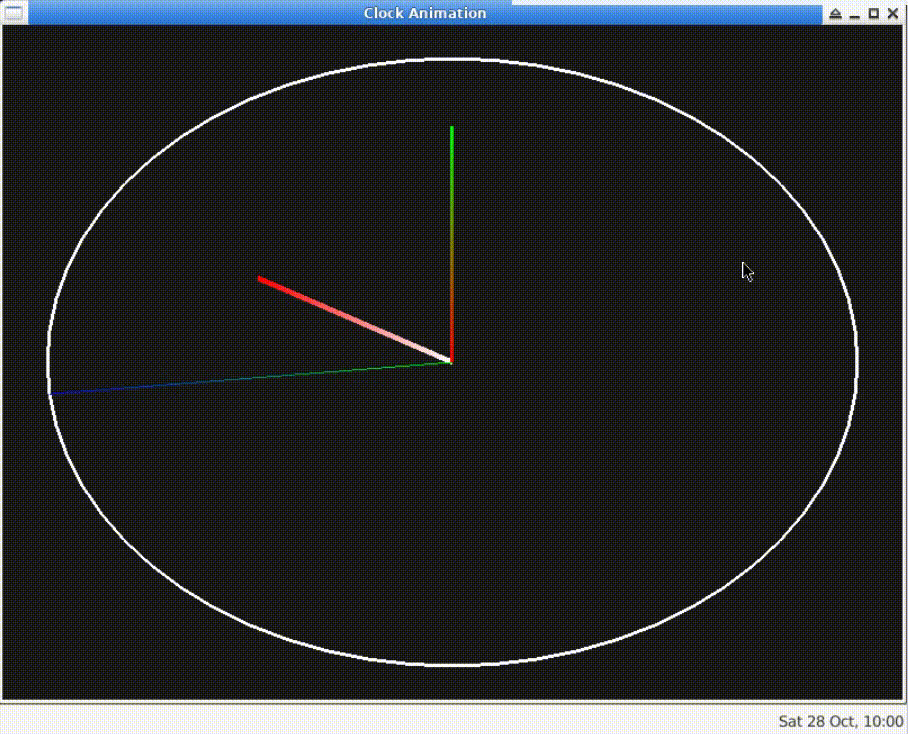
🎯 Tasks
In this project, you will learn:
- How to set up the project files and libraries
- How to create the window and initialize OpenGL
- How to draw the clock background and outline
- How to rotate the clock to have the 12 o'clock position at the top
- How to get the current time and calculate the positions of the clock hands
- How to draw the hour, minute, and second hands on the clock
- How to resize the window and display the clock in real-time
🏆 Achievements
After completing this project, you will be able to:
- Set up and initialize OpenGL and GLUT
- Draw basic shapes and lines using OpenGL
- Rotate objects in OpenGL
- Retrieve the current time and use it to animate objects
- Handle window resizing and real-time display of graphics