Introduction
In this project, we will create a simple text-based Gomoku game using the C programming language. Gomoku is a two-player strategy board game where the objective is to be the first to get five consecutive pieces in a row, either horizontally, vertically, or diagonally. We will develop this game using a 15x15
game board.
ð Preview
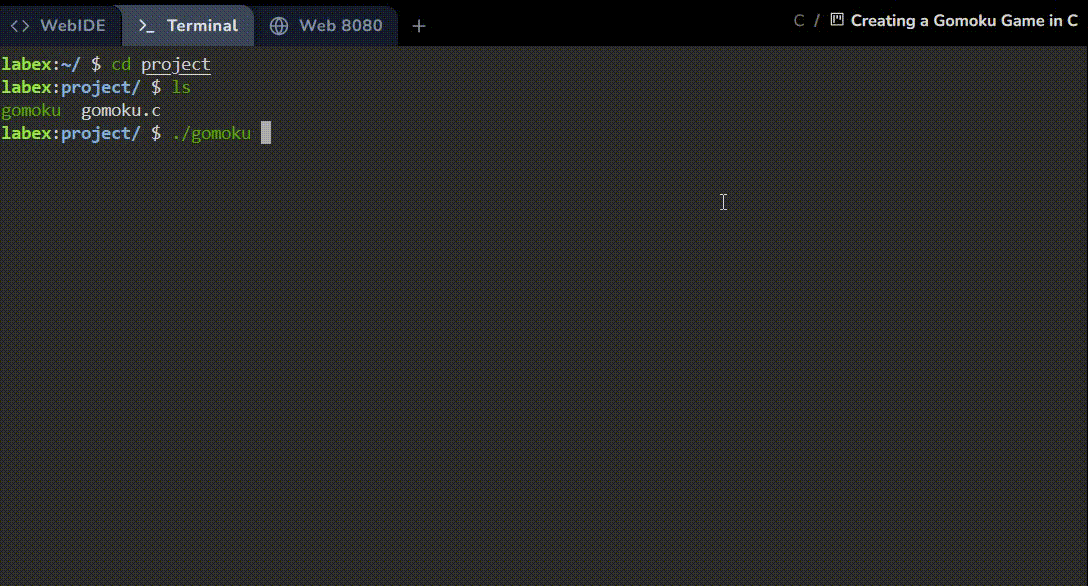
ðŊ Tasks
In this project, you will learn:
- How to design and implement a chessboard using a two-dimensional array in C.
- How to create a main function to control the flow of the game.
- How to implement functions to initialize the game, print the chessboard, and allow players to play their turns.
- How to develop a function to check for a winning condition in the game.
- How to compile and run the program.
ð Achievements
After completing this project, you will be able to:
- Work with two-dimensional arrays in C.
- Design and implement a game flow using functions.
- Check for winning conditions in a game.
- Compile and run a C program.