Introduction
In this project, you will learn how to create a simple code rain using the ncurses
library in the C programming language. Ncurses is a library that facilitates text-based user interfaces in the terminal. This project will guide you through setting up the project, initializing the necessary components, and implementing the code rain.
ð Preview
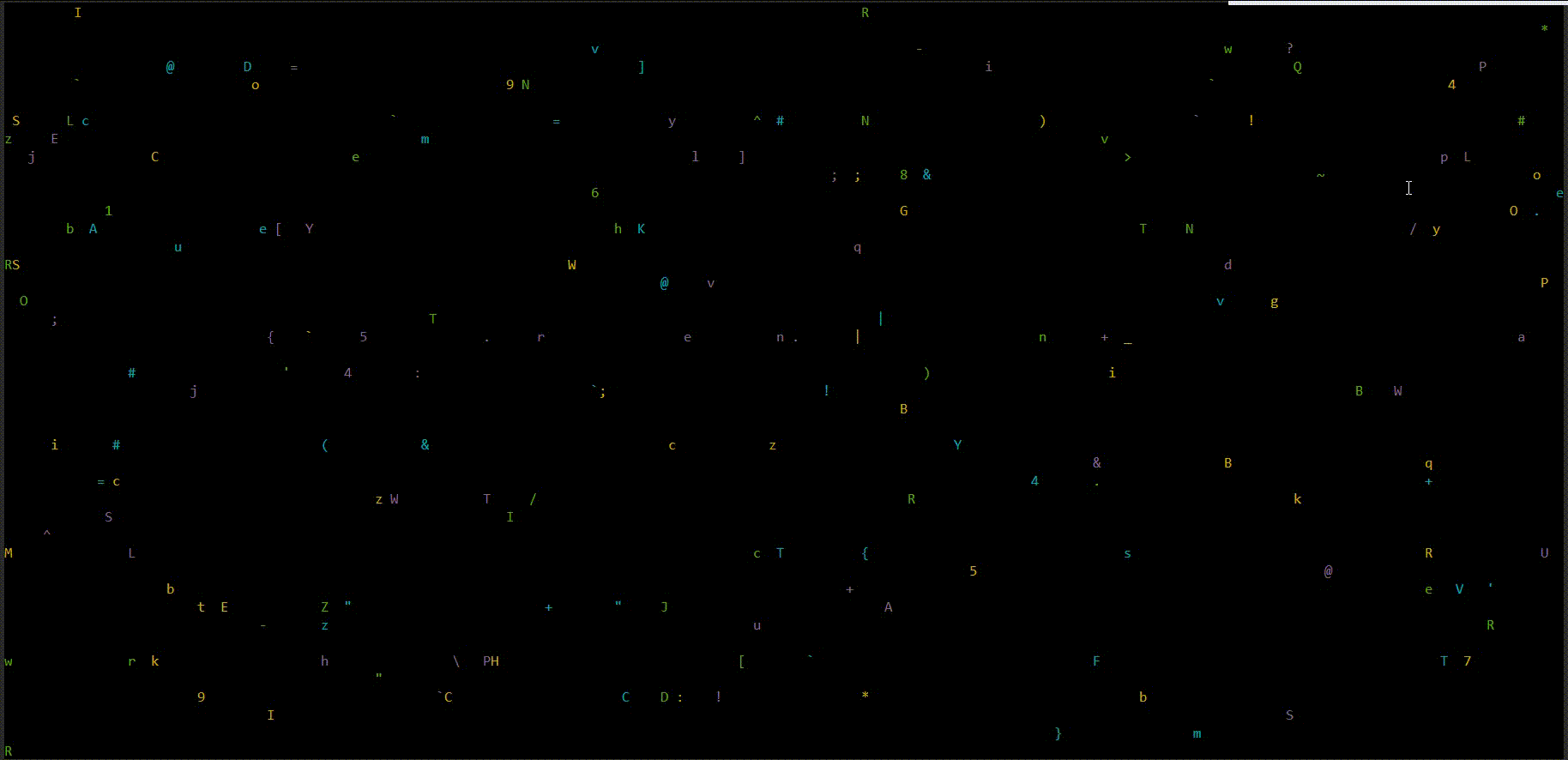
ðŊ Tasks
In this project, you will learn:
- How to install the ncurses library
- How to define constants and structures for the raindrops
- How to initialize colors for the animation
- How to create an animation loop to display the raindrops falling
- How to read user input to exit the animation
ð Achievements
After completing this project, you will be able to:
- Use the ncurses library in C
- Implement structures in C
- Create animations in the terminal