Introduction
In this project, you will learn how to use subqueries to retrieve relevant information from the employee (emp
) and department (dept
) tables in the personnel database. You will practice writing complex SQL queries to access and analyze data from multiple tables.
๐ Preview
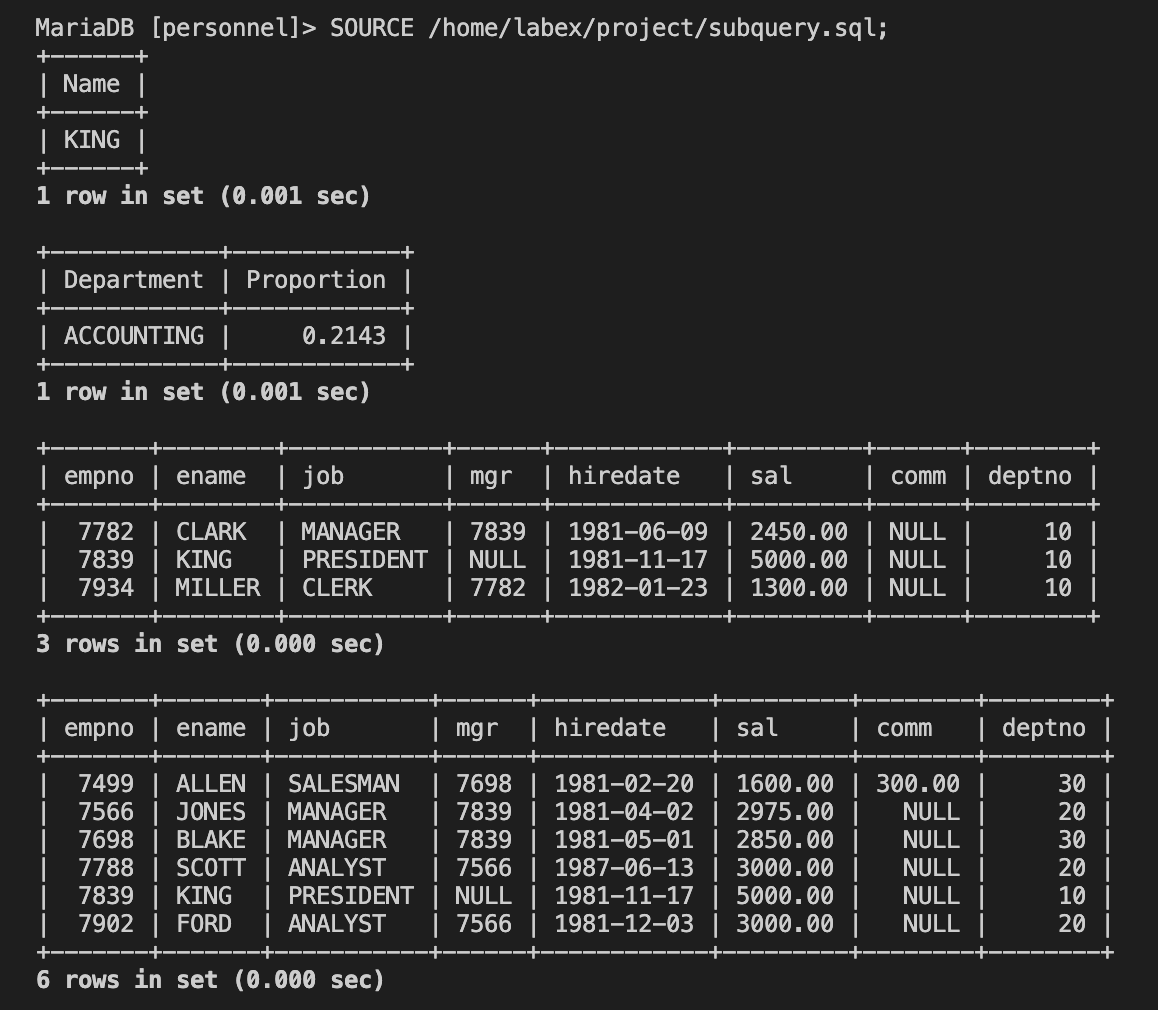
๐ฏ Tasks
In this project, you will learn:
- How to start the MySQL server and import the personnel database
- How to use a subquery to find the employee with the highest salary
- How to calculate the proportion of employees in a specific department compared to the entire company
- How to retrieve all employees working in a specific location using a subquery
- How to find employees whose salary exceeds the average salary in their department
๐ Achievements
After completing this project, you will be able to:
- Understand the concept and use of subqueries in SQL
- Write complex SQL queries that combine data from multiple tables
- Analyze and extract meaningful insights from a database using subqueries
- Demonstrate your SQL skills in a practical, real-world scenario