Introduction
In this project, you will learn how to implement navigation features in a React application. You will create a simple application with a navigation bar and pages that can be navigated to using links.
๐ Preview
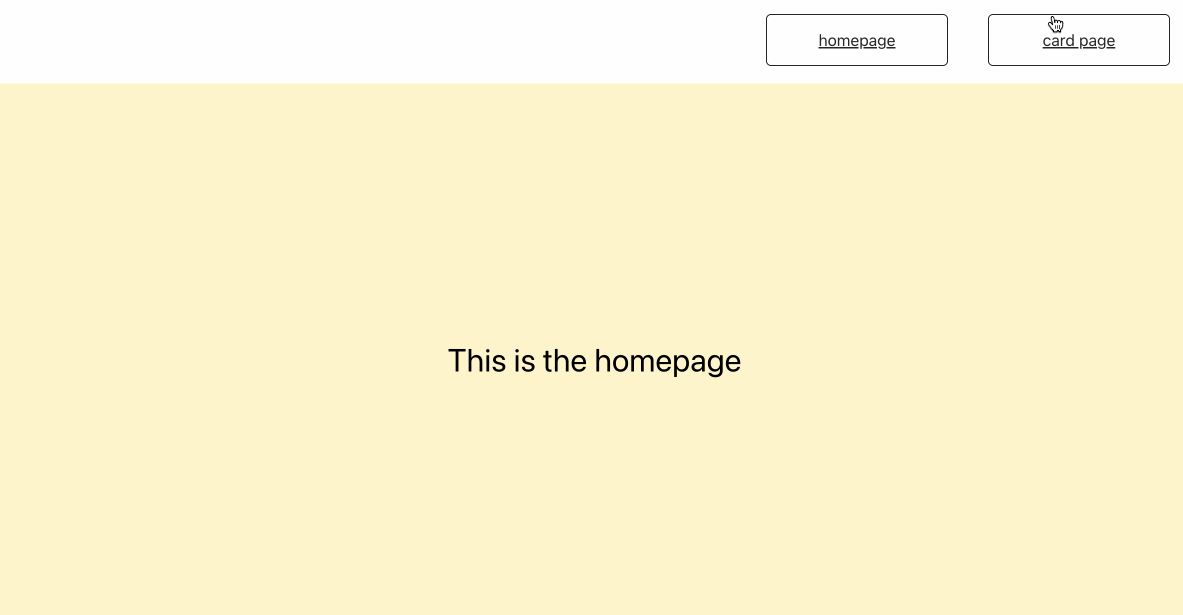
๐ฏ Tasks
In this project, you will learn:
- How to set up the project and install dependencies
- How to add routes and route matchers to enable navigation of the menus in the navigation bar
- How to add navigation from the card list to individual cards
๐ Achievements
After completing this project, you will be able to:
- Use React Router to handle client-side routing
- Create links and navigate between different pages in a React application
- Pass data between components using the
state
object in React Router