Introduction
In this project, you will learn how to create a simple racing game using the Pygame library. The game involves a player car and multiple enemy cars. The player car can be moved left and right using the arrow keys, while the enemy cars move down the screen. The goal is to avoid collisions with the enemy cars for as long as possible. The game will display a game over screen when a collision occurs and allow the player to restart the game by pressing the "R" key.
ð Preview
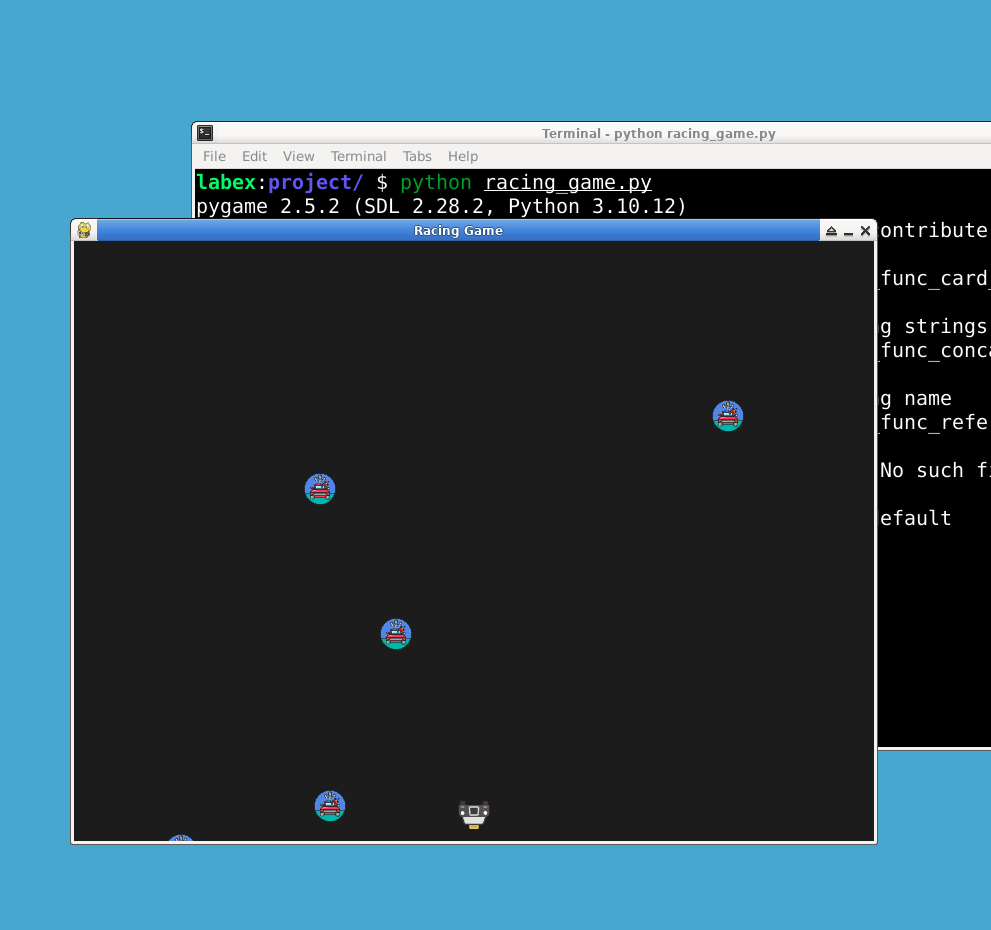
ðŊ Tasks
In this project, you will learn:
- How to set up the game window and import necessary libraries
- How to define colors and load car images
- How to define the player car
- How to define the enemy cars
- How to define game over variables and font
- How to implement the game logic
- How to display the game over screen
- How to quit the game
ð Achievements
After completing this project, you will be able to:
- Use the Pygame library to create a game window
- Load and display images in a game
- Handle collisions between game objects
- Implement a game loop for continuous gameplay
- Handle user input to control game objects
- Display text on the screen using Pygame's font module