Introduction
In this project, you will learn how to create a simple code rain animation using the Pygame library in Python. Code rain animations, popularized by movies like "The Matrix," display falling characters on a screen, giving the impression of a digital rain. We'll use the Pygame library to create the animation and display it on a window.
ð Preview
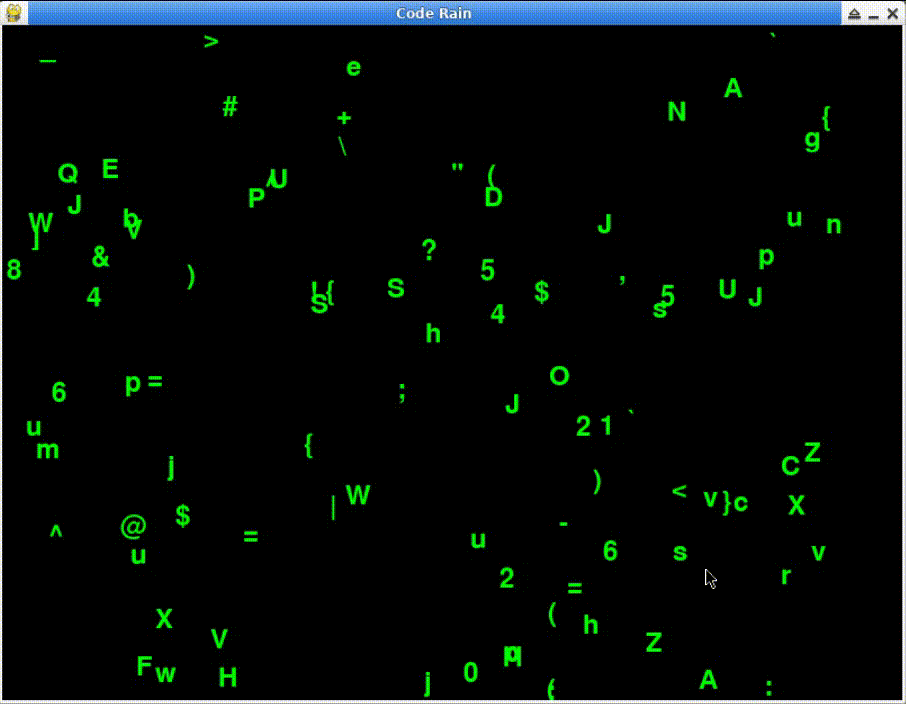
ðŊ Tasks
In this project, you will learn:
- How to set up the animation window in Pygame
- How to define a "Raindrop" class to represent each falling character
- How to generate and display multiple instances of the Raindrop class
- How to implement the main loop to continuously update the animation
- How to close the animation window properly
ð Achievements
After completing this project, you will be able to:
- Use the Pygame library to create animations
- Define and use a class in Python
- Handle user events in Pygame
- Create a main animation loop in Pygame
- Properly close an animation window in Pygame