Introduction
The Monty Hall problem is a famous probability puzzle based on a game show scenario. In the game, a contestant is presented with three doors. Behind one of the doors is a prize (like a car), while the other two doors hide goats. The contestant selects one of the doors. The host, who knows where the prize is, then opens one of the other two doors to reveal a goat. The contestant is then given the option to either stay with their original choice or switch to the other unopened door. The question is: What's the best strategy, to switch or to stay? This project will guide you through building a GUI application to simulate the Monty Hall problem using the Tkinter library in Python.
👀 Preview
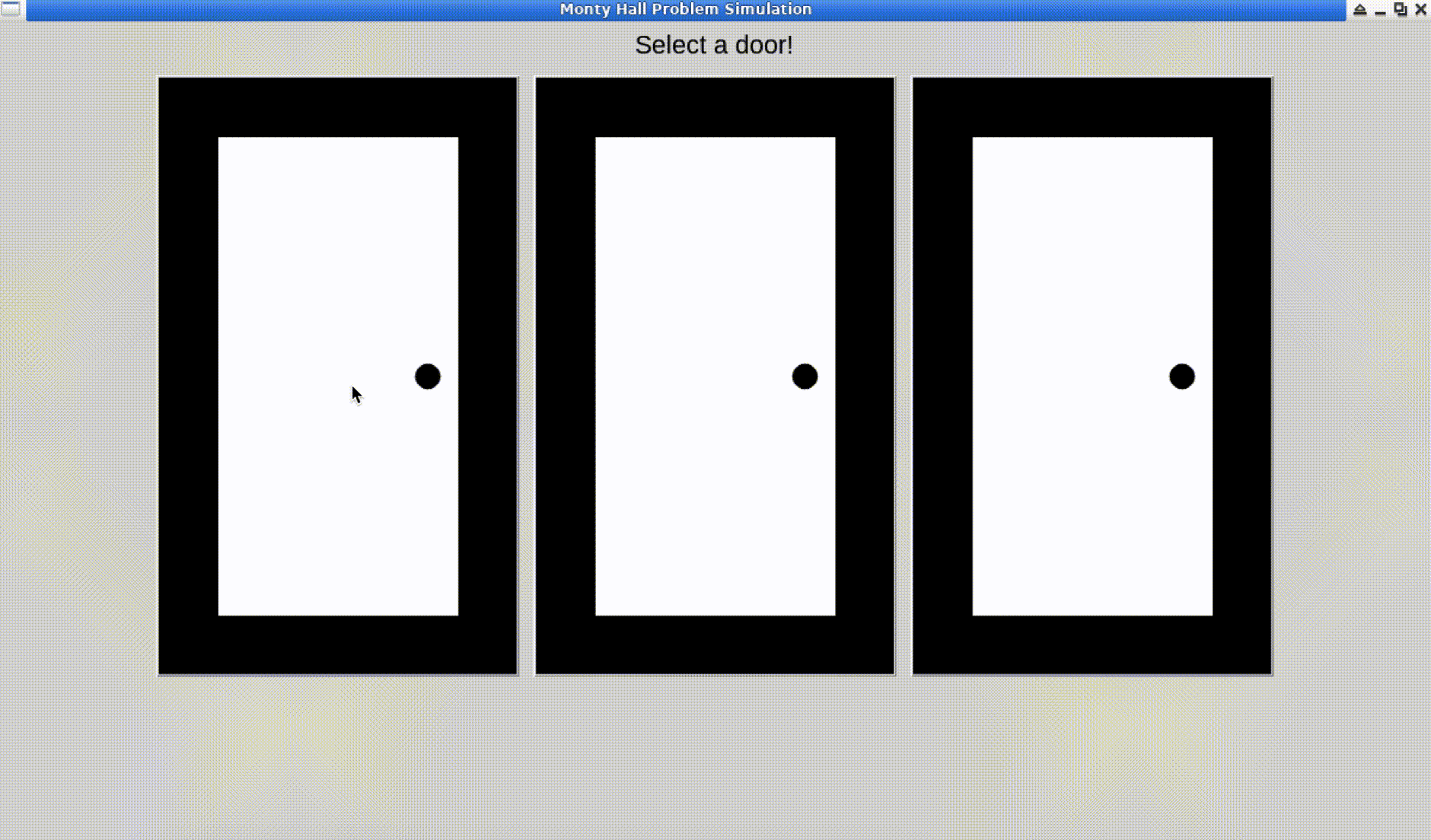
🎯 Tasks
In this project, you will learn:
- How to design and develop a graphical user interface (GUI) using Tkinter.
- How to simulate the Monty Hall problem to understand its probabilistic outcomes.
- How to implement game logic in Python to handle user choices and reveal results.
- How to use Python's random library to randomly allocate the prize behind one of the doors.
- How to reset game states to allow multiple rounds of play without restarting the application.
🏆 Achievements
After completing this project, you will be able to:
- Apply GUI design principles and implement them in Python with Tkinter.
- Understand the practical application of probability and statistics in game simulations.
- Implement event-driven programming and handle user interactions in a GUI application.
- Utilize advanced Python programming techniques such as lambda functions and list comprehensions.
- Recognize the importance of user experience (UX) in game design and provide feedback mechanisms using message boxes.