Introduction
In this project, you will learn how to build a network scanner using Python. The network scanner will utilize the nmap
command-line tool to scan a specified IP address and display the results in a graphical user interface (GUI) using the Tkinter library.
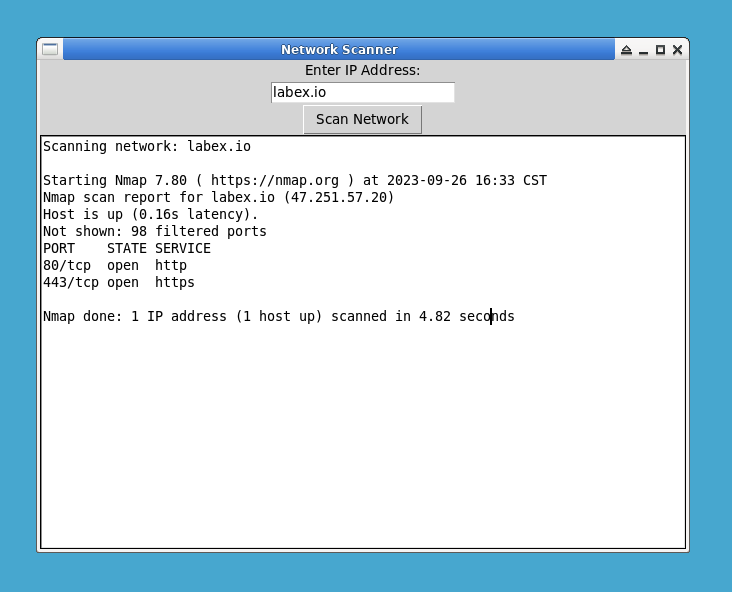
๐ฏ Tasks
In this project, you will learn:
- How to set up the project and install necessary libraries
- How to import necessary libraries for GUI and executing the
nmap
command-line tool - How to define a scanning function to retrieve the user-entered IP address and scan the network
- How to create the main window of the GUI
- How to add an input field for the IP address and a "Scan Network" button
- How to create a text area to display the scan results
- How to run the project and perform network scanning
๐ Achievements
After completing this project, you will be able to:
- Set up a Python project and install libraries
- Import libraries and use them in a Python script
- Create a GUI using Tkinter
- Handle user input and trigger functions
- Use the
nmap
command-line tool for network scanning