Introduction
In this project, you will learn how to create a Pong game using Python and the Pygame library. Pong is a classic two-player arcade game where players control paddles to hit a ball past each other.
To complete this project, you will need to follow the steps below. We will start by creating the project files and setting up the game window. Then, we will define the colors and set up the paddles and ball. Finally, we will move the paddles and ball, handle collisions, and draw the game elements.
ð Preview
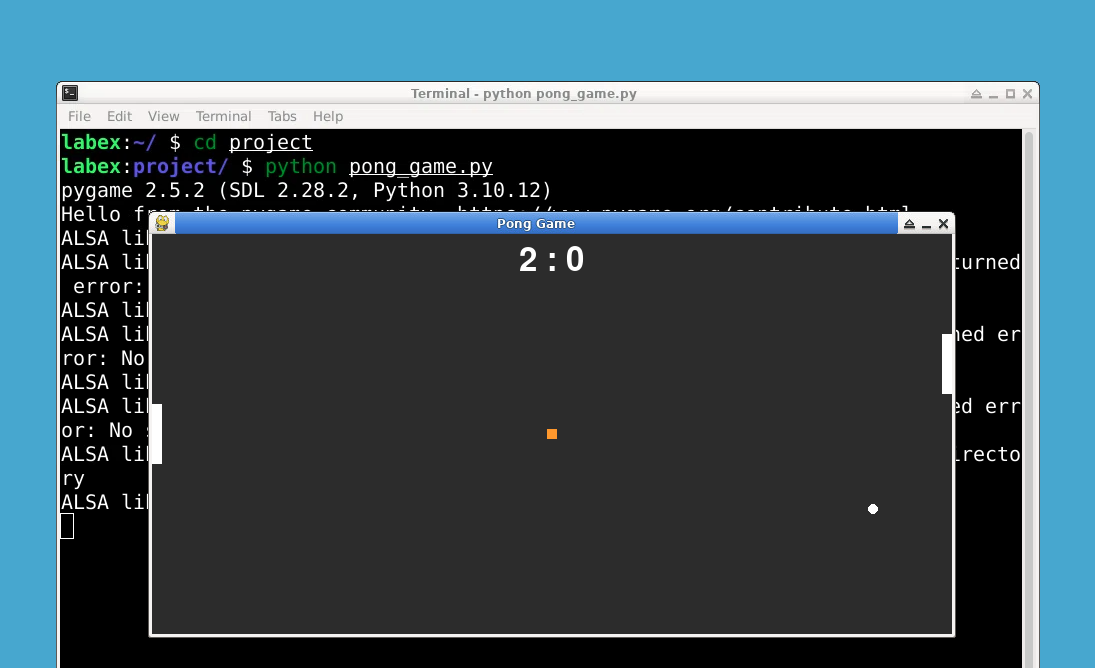
ðŊ Tasks
In this project, you will learn:
- How to create the project files
- How to set up the game window
- How to set up the paddles and ball
- How to set up the game variables
- How to set up the game loop
- How to move the paddles
- How to move the ball
- How to handle ball collisions
- How to update scores and reset the ball
- How to handle power-up collisions and movement
- How to draw the game elements
- How to draw the score
- How to update the display
- How to set the frames per second (FPS)
- How to quit the game
ð Achievements
After completing this project, you will be able to:
- Use the Pygame library to create a game window
- Set up and move game objects like paddles and a ball
- Handle collisions between game objects
- Update and display game scores
- Set the frames per second (FPS) for the game
- Quit the game properly