Introduction
In this project, you will learn how to create a university information query system using Java and MySQL. This project will guide you through the process of setting up a MySQL database, connecting to it using Java, and querying information about students, courses, and instructors.
👀 Preview
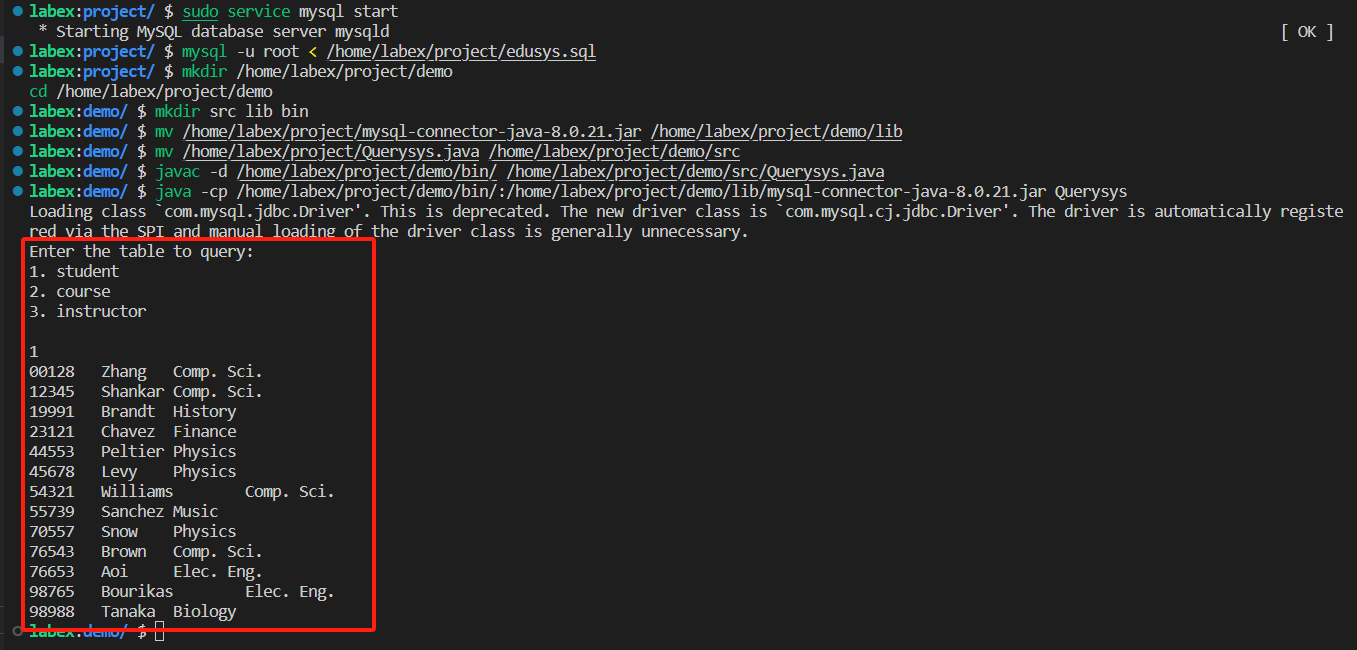
🎯 Tasks
In this project, you will learn:
- How to start the MySQL service and import a database
- How to create a Java project directory and organize your files
- How to connect to a MySQL database using Java and the JDBC driver
- How to write Java code to query information from the database and display the results
🏆 Achievements
After completing this project, you will be able to:
- Understand the basics of connecting a Java application to a MySQL database
- Write Java code to execute SQL queries and retrieve data
- Develop a simple information query system that can display data from a university database