Introduction
In this project, you will learn how to add student information to a MySQL database using Java programming. This project will guide you through the process of starting the MySQL server, importing a database script, creating a Java program to insert a new student record, and verifying the inserted record.
ð Preview
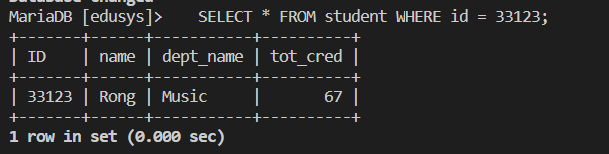
ðŊ Tasks
In this project, you will learn:
- How to start the MySQL server and log in to the MySQL command-line interface
- How to import a database script to create the necessary tables
- How to create a Java program to insert a new student record into the database using JDBC
- How to compile and run the Java program to insert the new record
- How to verify the inserted record in the MySQL database
ð Achievements
After completing this project, you will be able to:
- Understand the basic process of interacting with a MySQL database using Java
- Write Java code to execute SQL statements and insert data into a database
- Verify the correctness of the inserted data by querying the database
- Apply these skills to build more complex database-driven applications