Introduction
In this project, you will learn how to implement a user permission management system using JavaScript and jQuery. The project involves fetching user data asynchronously, rendering it in a user permission table, and providing functionality to move users between the user and administrator lists, as well as updating the user permissions accordingly.
👀 Preview
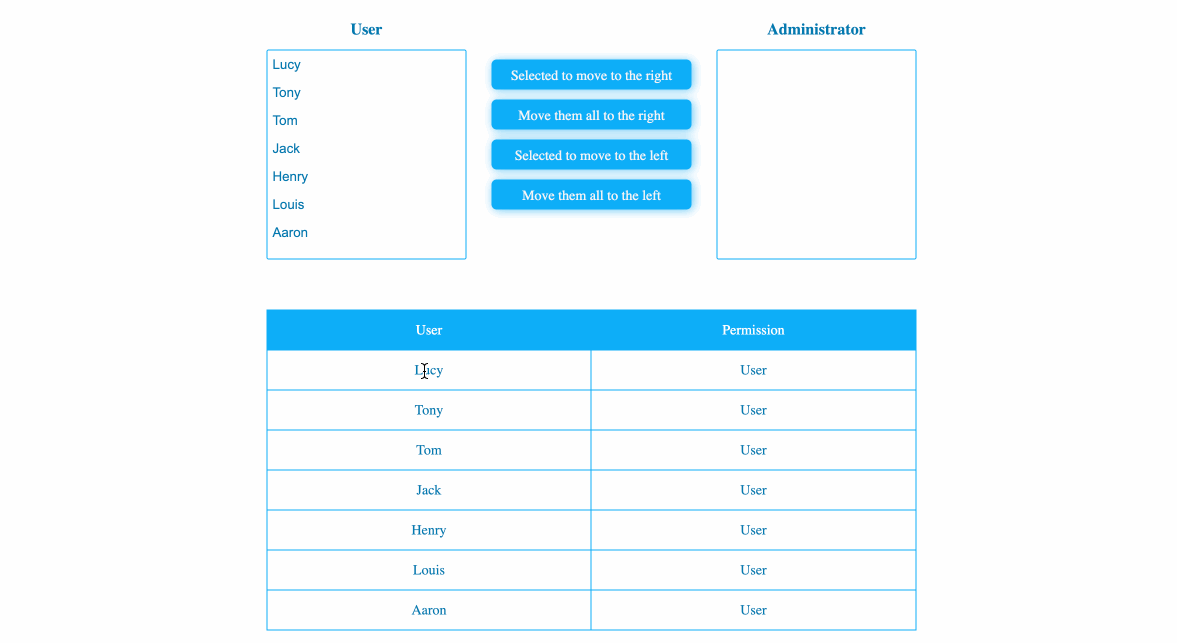
🎯 Tasks
In this project, you will learn:
- How to fetch user data asynchronously from a JSON file and render it in a user permission table
- How to implement the functionality to move users between the user and administrator lists, both individually and all at once
- How to update the user permissions in the user permission table based on the users in the left and right lists
🏆 Achievements
After completing this project, you will be able to:
- Use AJAX to fetch data asynchronously
- Manipulate the DOM using jQuery to update the user interface
- Implement permission management functionality in a web application