Quick Start with Shell

Our Quick Start with Shell course offers a solid foundation in Bash shell scripting, covering Linux command-line essentials. Through hands-on labs and real-world challenges, you'll master file management, text processing, and automation techniques, enabling you to efficiently use the shell in your daily tasks.
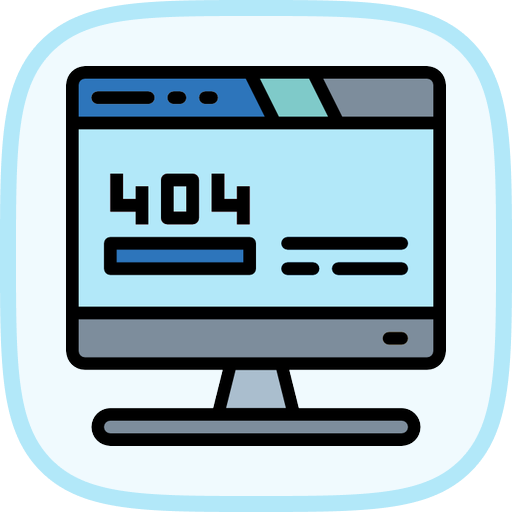
Hello, Bash!
Dive into the world of shell scripting with this hands-on lab. Learn to create, edit, and execute a simple Bash script that prints the classic 'Hello, World!' message. Master essential Linux commands and Bash scripting fundamentals along the way.
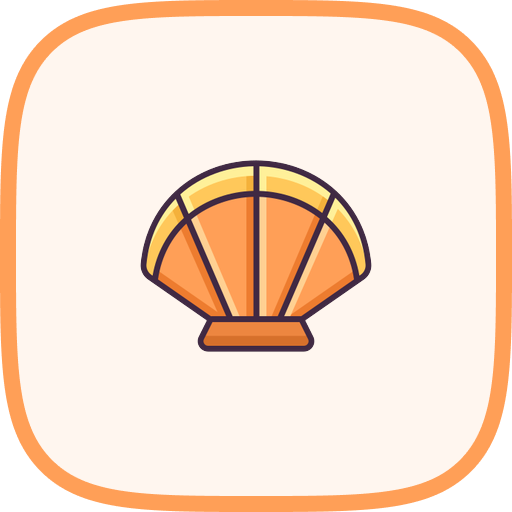
Working with Shell Variables
Learn the fundamentals of shell variables in this hands-on lab. Learn how to create, reference, and manipulate variables, perform command substitution, execute arithmetic operations, and work with environment variables in shell scripts.
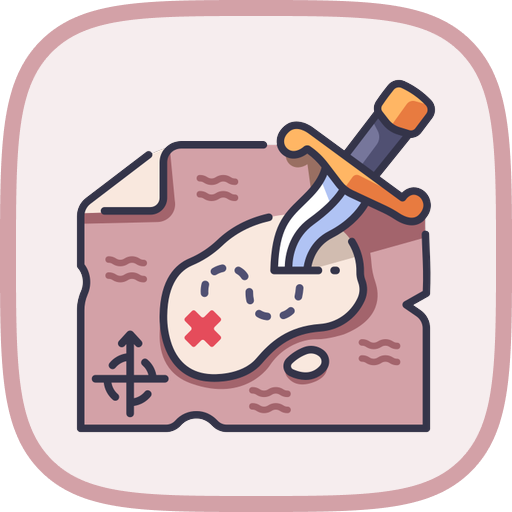
Finding the Pirate's Treasure
Learn shell scripting basics by decoding Captain Blackbeard's treasure map using shell variables and arithmetic operations. Practice creating executable scripts, using variables, and performing arithmetic calculations in Bash.
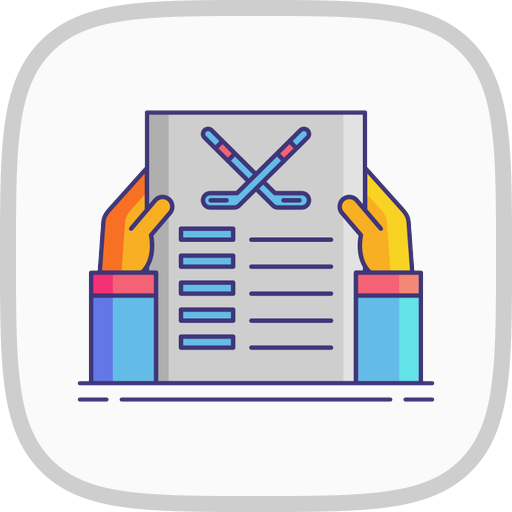
Passing Arguments to the Script
Learn the art of passing and handling arguments in Shell scripts. This hands-on lab will guide you through creating a script that accepts command-line arguments, accessing them using special variables, and implementing logic based on the number and content of these arguments.
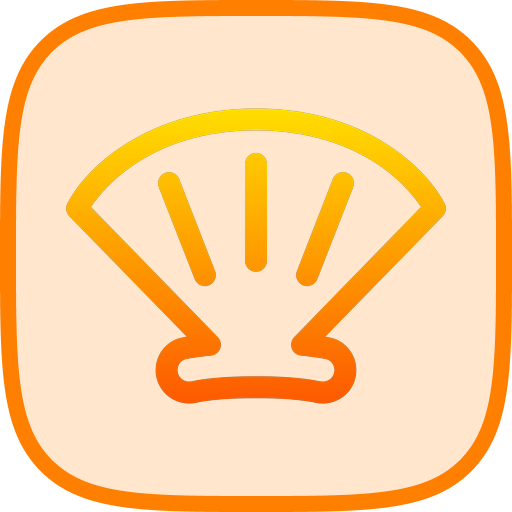
Shell Arrays
Explore the power of arrays in shell programming through this hands-on lab. Learn to create, manipulate, and access elements in arrays, enabling efficient data organization and manipulation in your shell scripts.
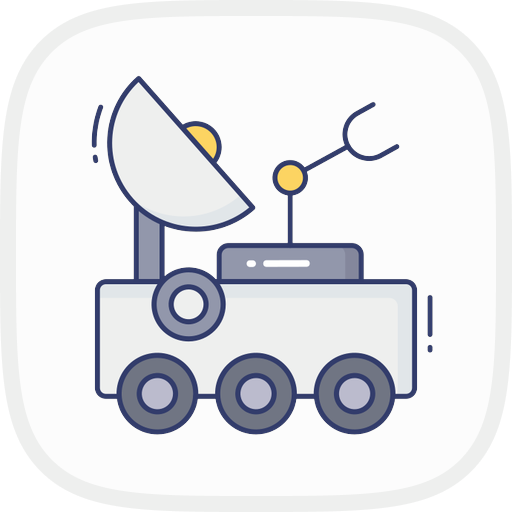
Interstellar Cargo Manifest
Welcome, space cadet! As a trainee cargo officer on the interstellar ship 'Nebula Nomad,' your task is to create a simple inventory system for the ship's three cargo bays. You'll write a shell script to manage and display the contents of each bay, handling different user inputs and providing appropriate responses.
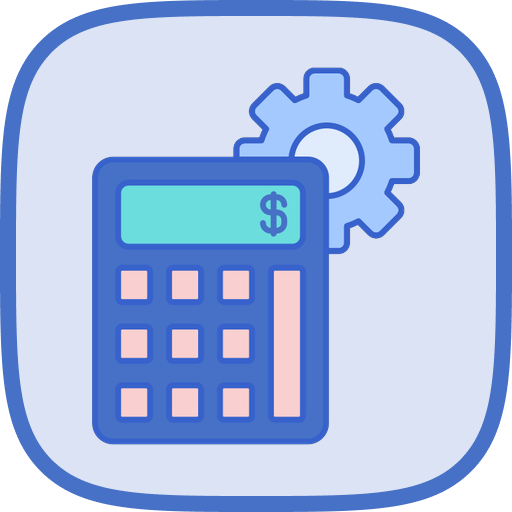
Arithmetic Operations in Shell
Learn arithmetic operations in Shell programming through this hands-on lab. Learn to perform calculations, use variables, and employ basic arithmetic operators to solve real-world problems like calculating costs in a shell script.
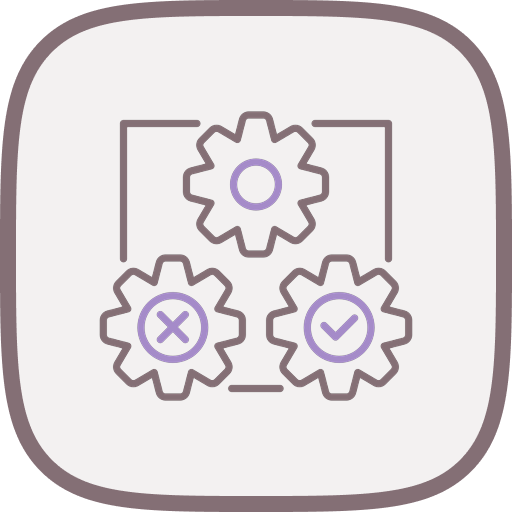
Basic String Operations
Dive into essential string manipulation techniques in shell scripting. This hands-on lab covers string length calculation, character position finding, substring extraction, and string replacement, providing you with practical skills for text processing in shell scripts.
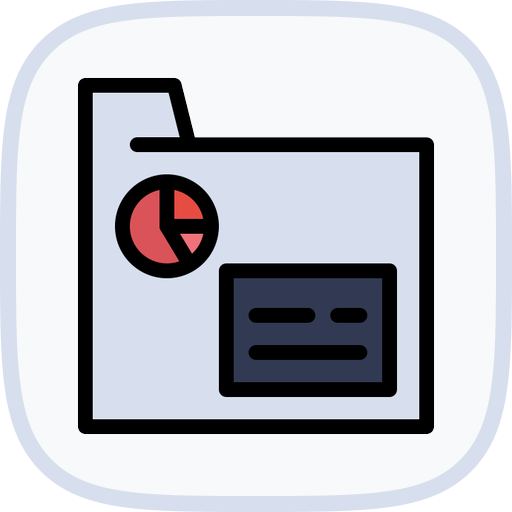
Conditional Statements in Shell
In this lab, you will learn how to use conditional statements in shell programming to make logical decisions. You'll practice writing if-else statements, using elif for multiple conditions, performing numeric and string comparisons, and combining conditions with logical operators. By the end of this lab, you'll be able to create shell scripts that can make decisions based on various conditions.
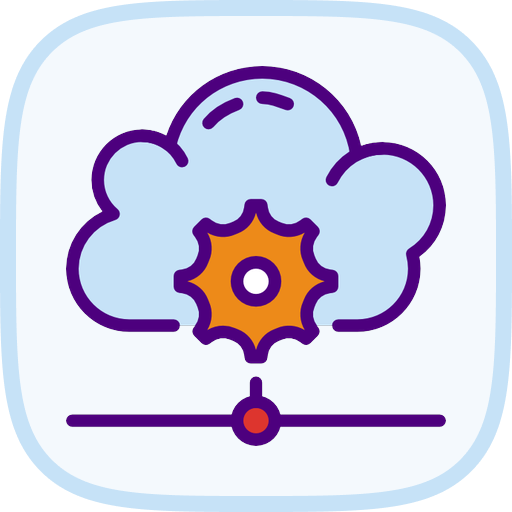
Weather Advisory System
In this challenge, you'll develop a simple weather advisory system for a local meteorology office. Your task is to create a shell script that provides different advice based on the current temperature. This exercise will help you practice using conditional statements in bash scripting to handle various scenarios.
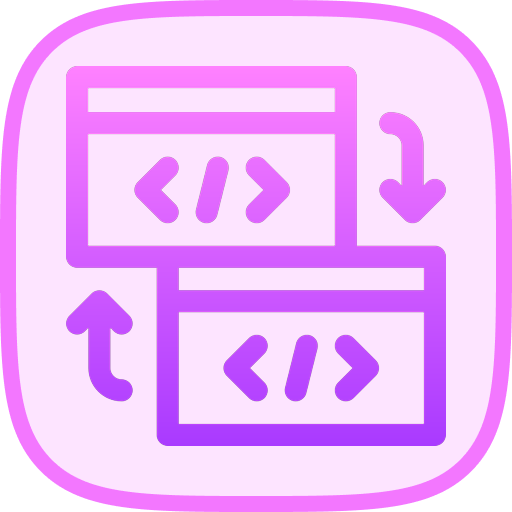
Bash Scripting Loops
In this lab, you will master the use of loops in Bash scripting. You'll learn how to implement for, while, and until loops to efficiently repeat instructions. Additionally, you'll explore break and continue statements to control loop execution. By the end of this lab, you'll be able to create more dynamic and flexible Bash scripts using various loop structures.
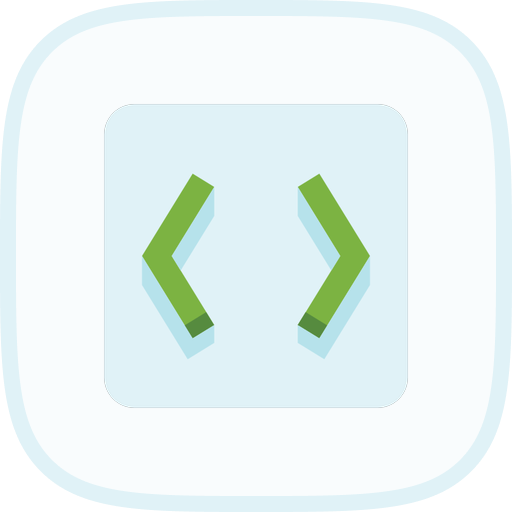
Comparing Arrays in Shell
In this lab, you will learn how to compare arrays in Shell scripting. You'll work with three arrays and implement a script to find common elements among them. This exercise will enhance your understanding of array manipulation, loops, and conditional statements in shell scripting.
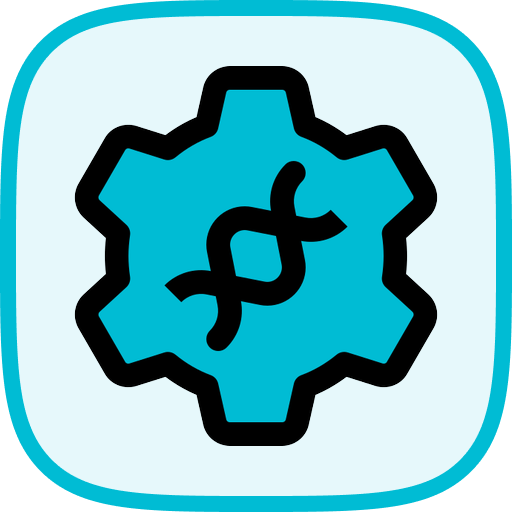
Shell Functions
In this lab, you will learn about functions in shell programming. You'll create and use functions with parameters, explore return values, understand variable scope, and implement an advanced function. By the end of this lab, you'll be able to write reusable code blocks to perform repeated tasks efficiently in shell scripts.
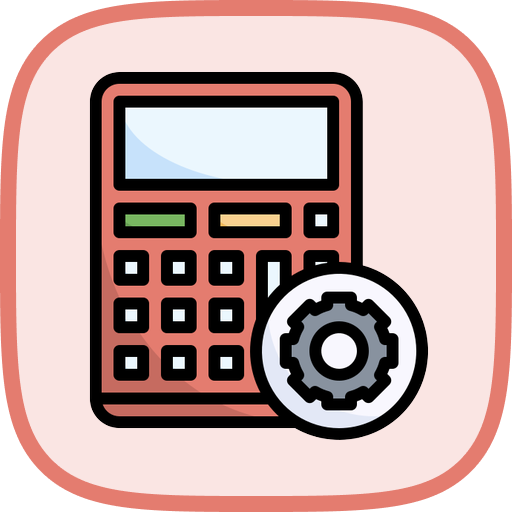
Four Function Calculator
In this challenge, you'll create a basic four-function calculator using shell scripting. You'll implement functions for addition, subtraction, multiplication, and division, handling various scenarios including division by zero. This exercise will help you practice function definition, arithmetic operations, and error handling in bash scripting.
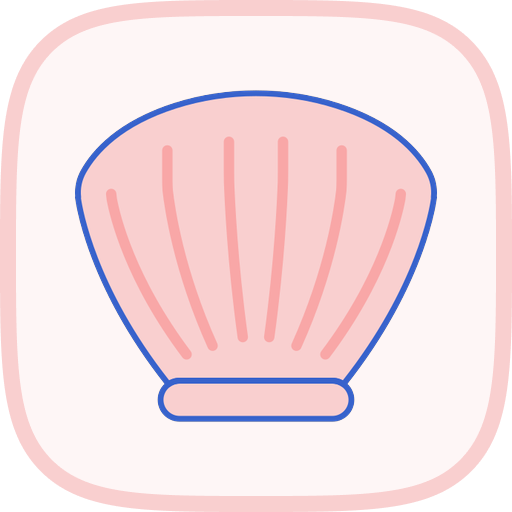
Special Variables in Shell
In this lab, you will explore special variables in shell scripting. You'll learn to use variables like $0, $1, $#, $?, and $$ to access script name, command-line arguments, argument count, exit status, and process ID. You'll also understand the difference between $@ and $* for handling multiple arguments.
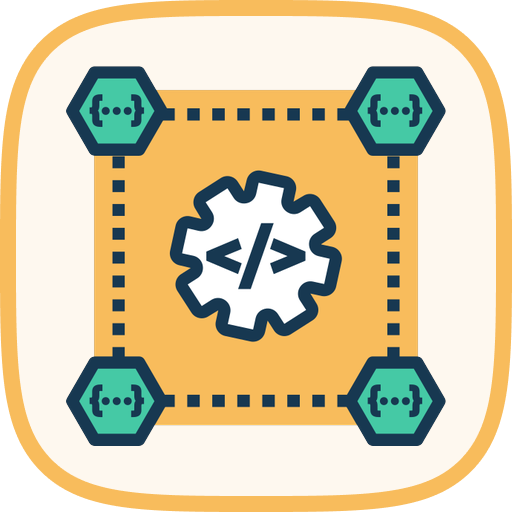
Bash Trap Command
In this lab, you'll learn to use the Bash trap command to handle signals and interruptions in your scripts. You'll create a script that catches specific signals, implement custom actions for these signals, and use functions with trap for more organized code. By the end, you'll be able to write scripts that gracefully handle various scenarios and prevent unpredictable behavior.
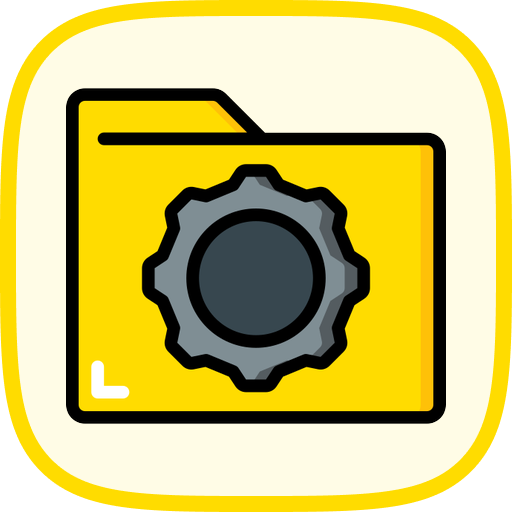
File System Operations in Shell
In this lab, you will learn how to perform various file tests in the shell. You'll create test files and directories, check for their existence, and test file permissions. By the end of this lab, you'll be able to write shell scripts that can interact with the file system and make decisions based on file properties.
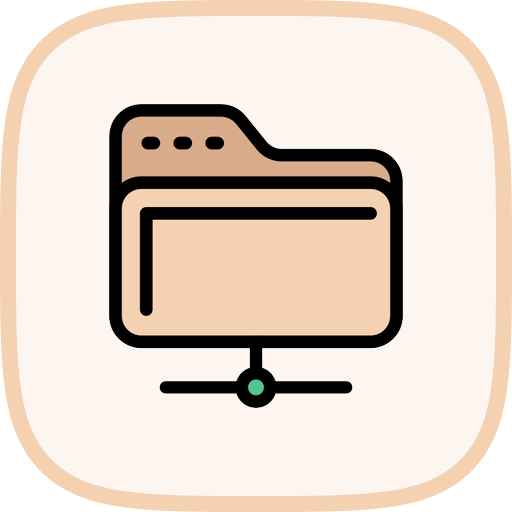
File System Explorer
In this challenge, you'll create a simple file system explorer script that demonstrates your understanding of basic file and directory operations in shell scripting. You'll implement functions to check the existence, type, and permissions of files and directories, enhancing your skills in file system manipulation and conditional testing in bash.