Quick Start with Java

Java is one of the most popular and powerful programming languages. It will walk you through the basic need-to-knows about Java. If you're new to this language or you want to refresh your memory, this is a great place to start. After learning this course, you will be able to learn to build advanced Java projects. Come on!
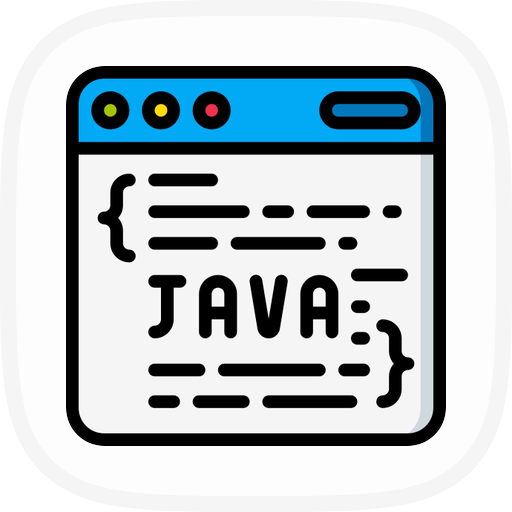
Introduction to Java Programming
Discover the fundamentals of Java programming in this beginner-friendly lab. Learn about high-level programming languages, Java's advantages, and create your first Java program from scratch to execution.
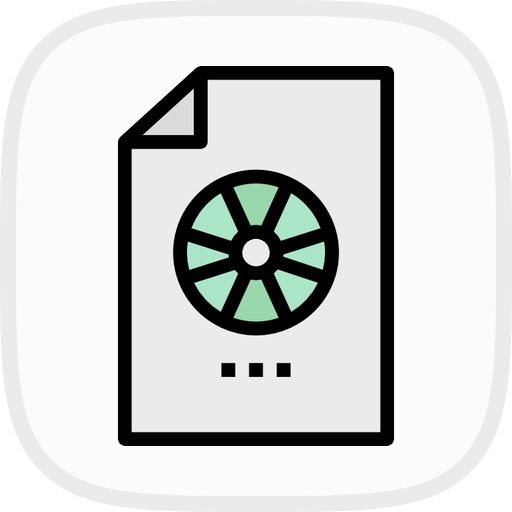
Simple Java Print Statement
In this challenge, you'll practice using the `System.out.println` statement in Java
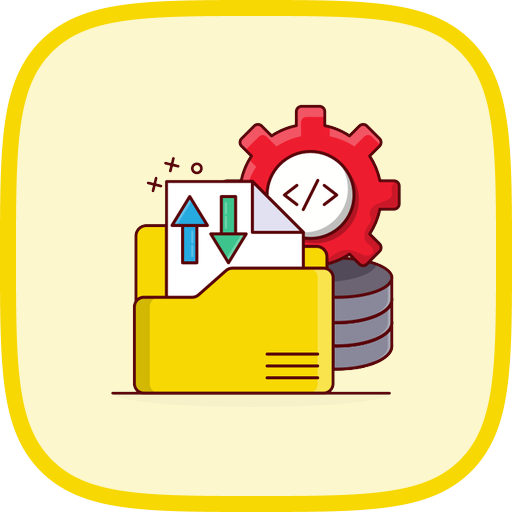
Variables and Operators in Java
Master Java variables, data types, and operators in this hands-on lab. Learn to declare variables, work with primitive data types, and apply arithmetic, bitwise, and logical operators in Java programming.
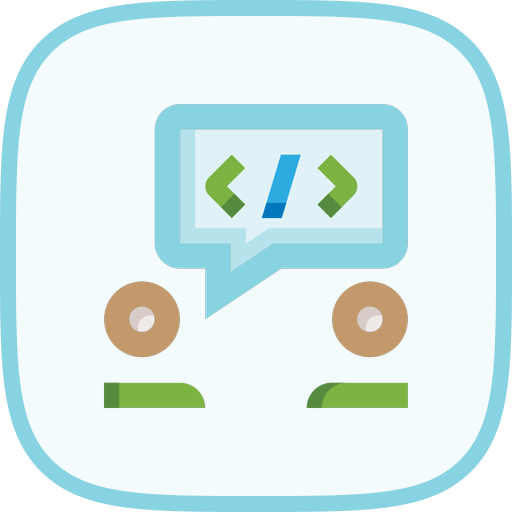
Java Conditional Expressions Fundamentals
In this lab, we learned basic data types of Java and operators. In this lab, we'll begin to learn to write procedure-oriented programs. The main idea is to use paradigm of controlling structures: conditional expressions.
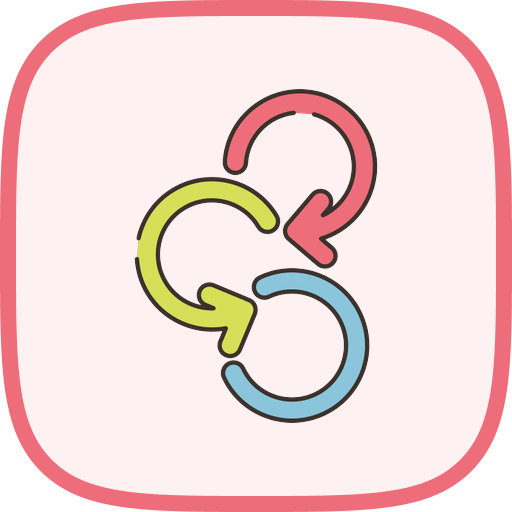
Recursion and Loops
Computers are often used to automate repetitive tasks. Repeating tasks without making errors is something that computers do well and people do poorly. Like conditional expressions, loops can also be nested one in another, but this will make our program hard to read and the performance will not be good. Try to avoid nested loops. There are three types of loops in Java. We'll learn them one by one in this lab.
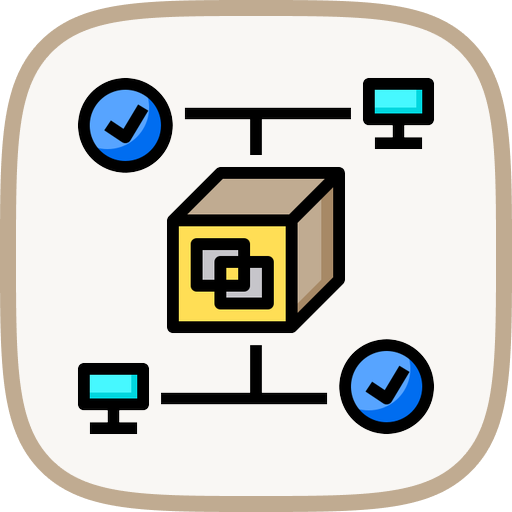
Methods & Parameters and Object
In this lab, we move forward to methods and objects. The task is to learn how to define a method with parameters. Object is a very important concept in OOP language, so having a good knowledge of it will be good for you.
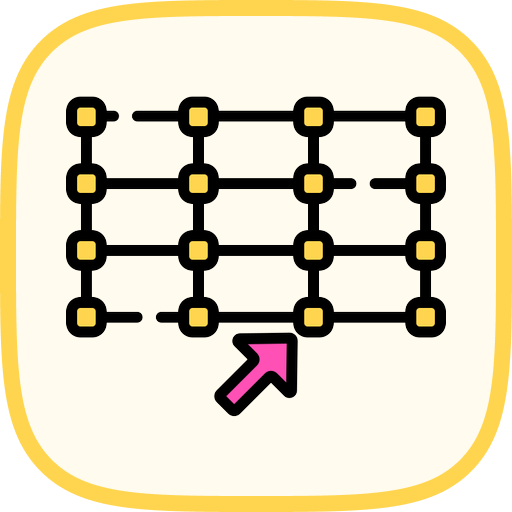
Number, String and Array
In Java and other object-oriented languages, objects are collections of related data that come with a set of methods. These methods operate on the objects, performing computations and sometimes modifying the objectโs data. Here we introduce three simple but important Java built-in object types: Number, String and Array.
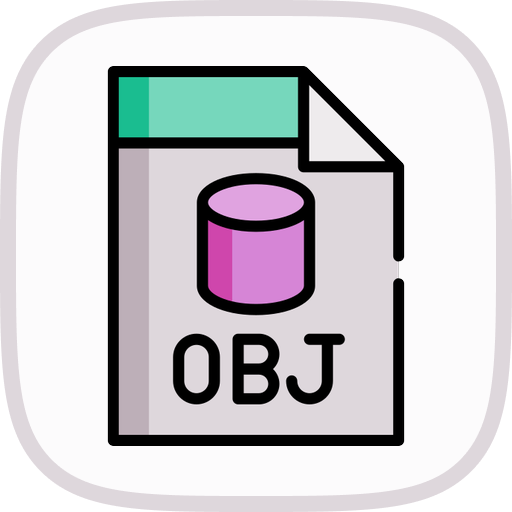
Class and Object
In this lab, you are going to learn a very important programming pattern: the object-oriented-programming. You need to know the difference between class and object. Take more practice to get a better understanding.
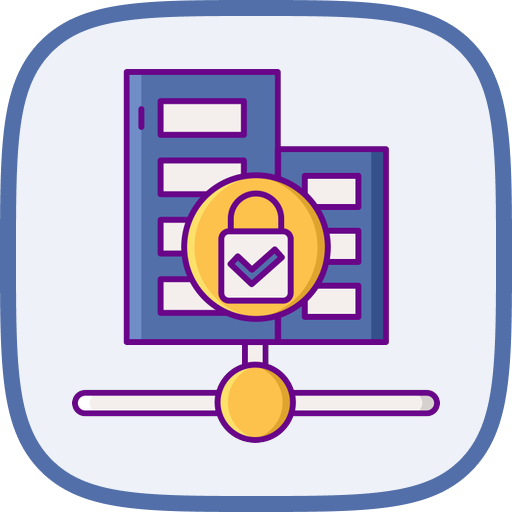
Access Modifiers and Inheritance
In this lab, you will learn access modifiers and inheritance. with different modifiers, access levels are different. Inheritance in Java is like biological inheritance in that children can keep parent's features and behave differently in some way.
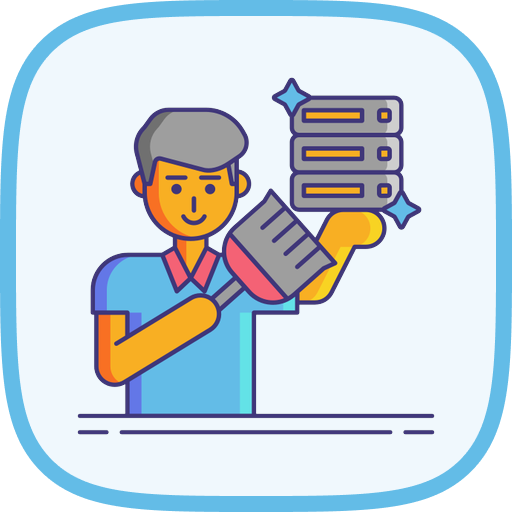
Overloading and Overriding
In this lab, you will learn method overloading and method overriding. Overriding and overloading are two concepts used in Java programming language. Both the concepts allow programmer to provide different implementations for methods under the same name. Overloading happens at compile-time while overriding happens at runtime. Static methods can be overloaded but cannot be overridden. Overloading is a static bond while overriding is dynamic bond.
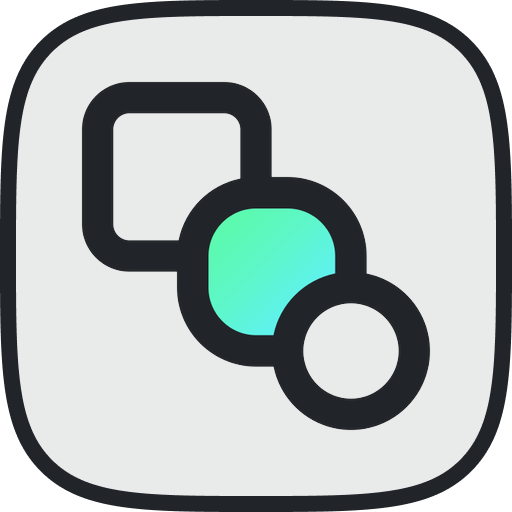
Abstraction and Interface
Abstraction and interface are two other significant concepts in Java. Abstract class and interface are very different although there are some common points. Start this lab and comprehend the concepts.
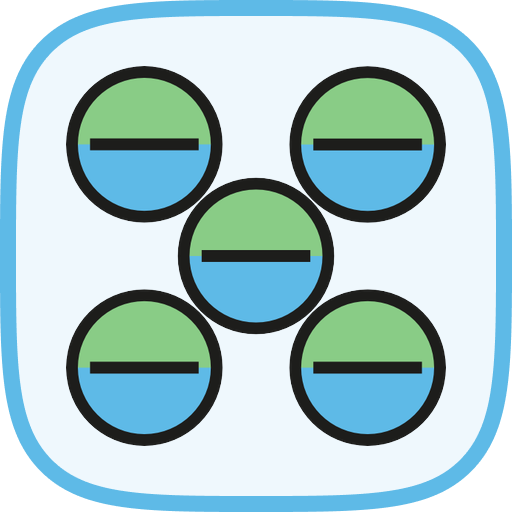
Polymorphism and Encapsulation
Encapsulation is like a bag that encloses the operations and the data related to an object together. Polymorphism is the ability of an object to take on many forms. In this lab, you will see what they are like.
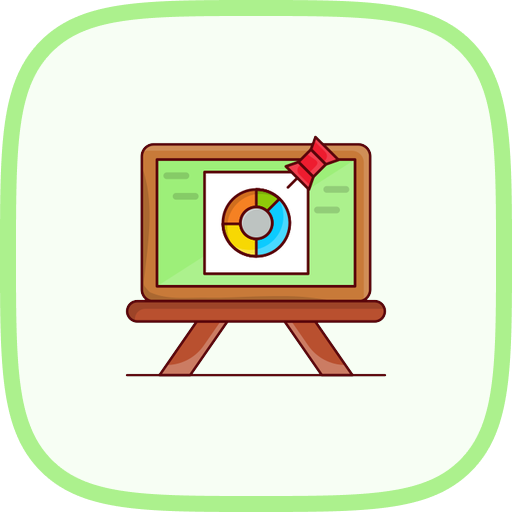
Arranging Classes by Functionality
In this lab, you will learn to use packages to arrange for classes according to their functionalities.