Introduction
In this project, you will learn how to generate a battle report based on the data files provided. The battle report will summarize the key information from the battles that occurred at three different enemy bases, including the battle ID, value, total participants, injured, dead, and fallen heroes.
ð Preview
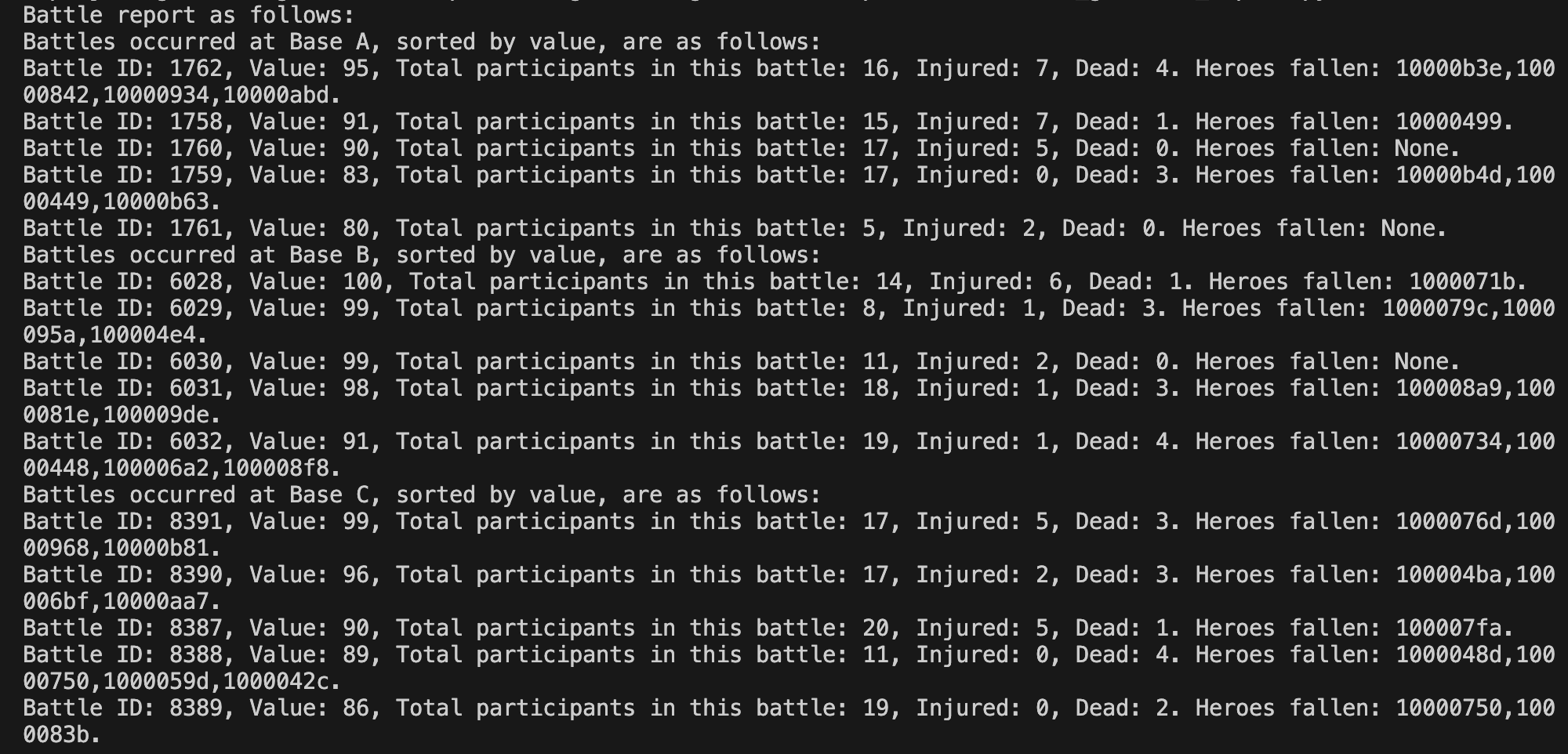
ðŊ Tasks
In this project, you will learn:
- How to read and understand the data files containing the battle information
- How to implement the
auto_generate_report
function to generate the battle report - How to enhance the battle report with additional formatting and information
- How to test and refine the battle report to ensure it meets the requirements
ð Achievements
After completing this project, you will be able to:
- Efficiently read and process data from CSV files
- Implement a function to generate a structured and informative report
- Demonstrate your ability to follow step-by-step instructions and complete a coding project
- Enhance your skills in data manipulation, formatting, and reporting