Introduction
In this project, we will guide you on how to create a basic GUI calculator using Python and the tkinter library. This calculator will be able to perform simple arithmetic operations such as addition, subtraction, multiplication, and division.
ð Preview
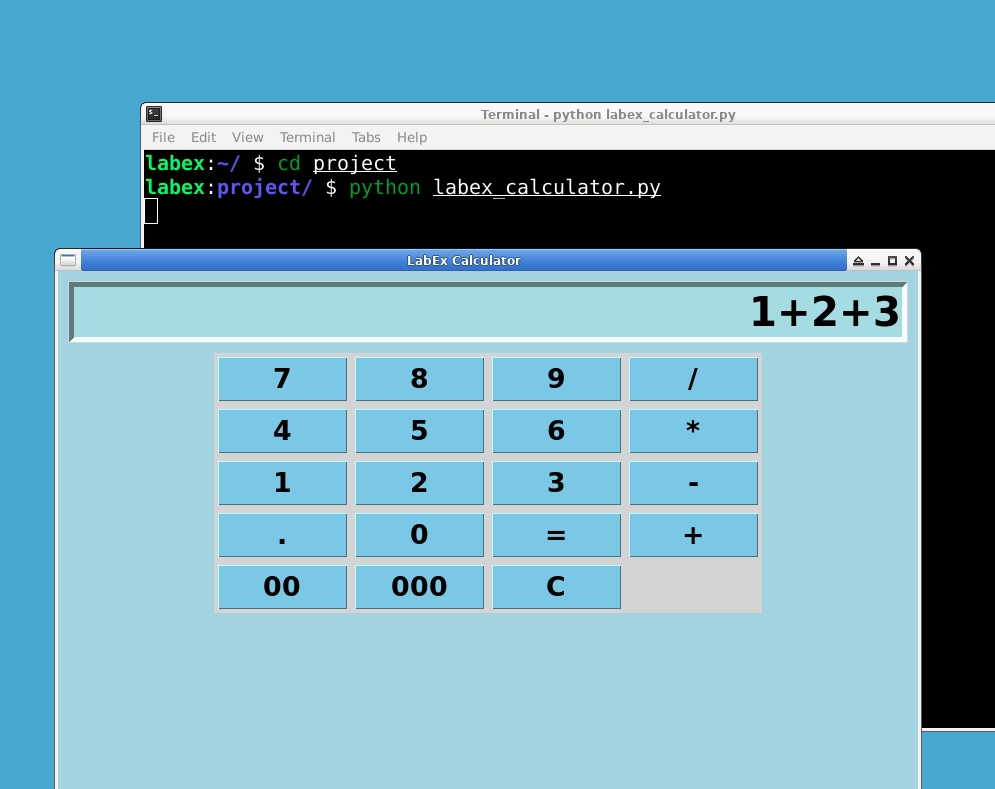
ðŊ Tasks
In this project, you will learn:
- How to set up the main tkinter window for the calculator
- How to add a calculation function to perform arithmetic operations
- How to create an entry field for users to input numbers and view results
- How to add buttons for digits, arithmetic operations, and clear function
- How to run the tkinter event loop to start the calculator
ð Achievements
After completing this project, you will be able to:
- Use the tkinter library to create a graphical user interface
- Bind functions to buttons to enable interactivity
- Perform basic arithmetic operations in Python
- Display and update results using an entry field