Introduction
In this project, we will create a digital clock application using Python and the Tkinter library. This clock will display both the current time and date, and it will allow the user to toggle between 12-hour and 24-hour time formats. We will split the code into multiple steps, starting with installing the required libraries and ending with running the project.
ð Preview
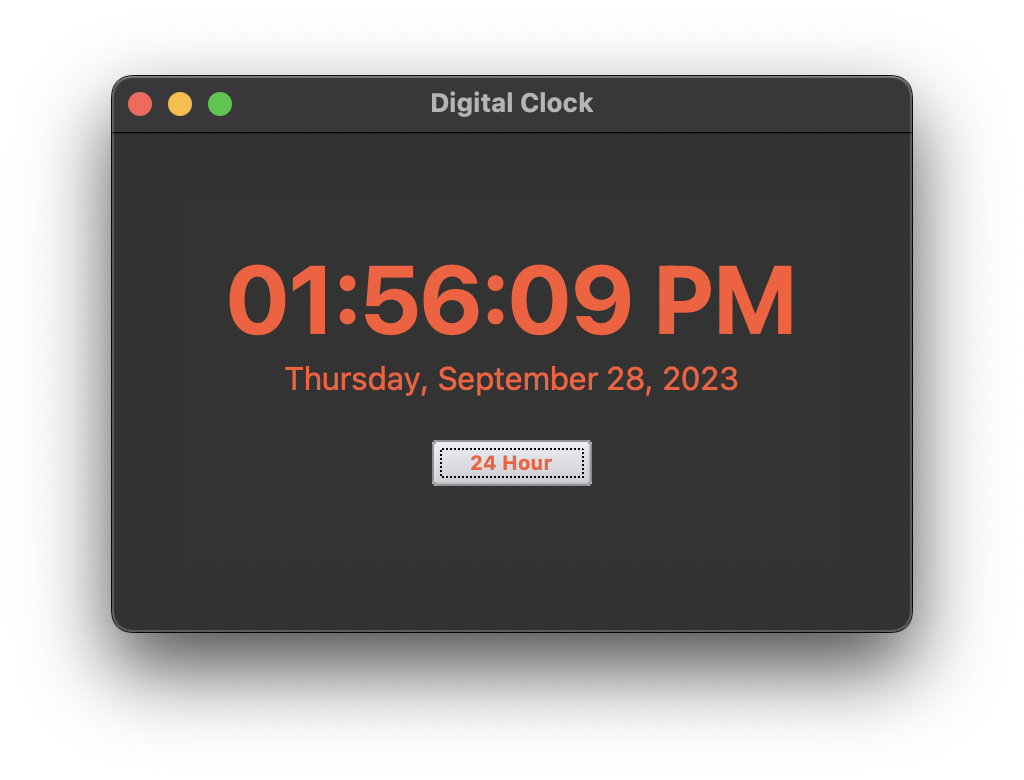
ðŊ Tasks
In this project, you will learn:
- How to install the necessary libraries for building a digital clock with Python.
- How to set up the project by creating the Python script and importing the required libraries.
- How to define a function to update and display the current time and date.
- How to create a function to toggle between 12-hour and 24-hour time formats.
- How to create the main application window and configure GUI styles.
- How to create and place the GUI components on the main window.
- How to start the clock by calling the necessary functions and running the main GUI loop.
ð Achievements
After completing this project, you will be able to:
- Install libraries using pip, the Python package manager.
- Use the Tkinter library to create graphical user interfaces in Python.
- Format and display time and date in Python.
- Customize the appearance of GUI components using themed styles.
- Create buttons with different text and functionality.
- Run a Python script and execute a main GUI loop.