Introduction
In this project, you will learn how to use JDBC (Java Database Connectivity) to query data from a MySQL database using the Statement
object. You will also learn how to encapsulate the result set using ResultSet
.
ð Preview
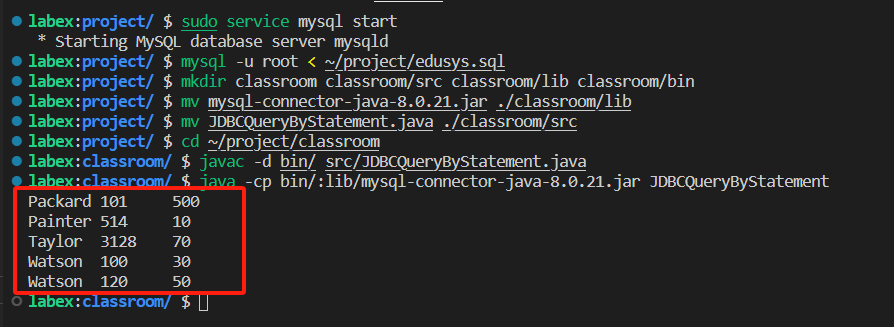
ðŊ Tasks
In this project, you will learn:
- How to start the MySQL service and import data into the database
- How to create a new Java project directory and move the necessary files
- How to implement the JDBC query using the
Statement
object - How to compile and run the Java application
ð Achievements
After completing this project, you will be able to:
- Establish a connection to a MySQL database using JDBC
- Create a
Statement
object to execute SQL queries - Encapsulate the result set using
ResultSet
- Retrieve and display data from a MySQL database