Introduction
In this project, you will learn how to add various constraints to a database schema, including primary keys, foreign keys, unique constraints, default values, and check constraints. By the end of this project, you will have a better understanding of how to design and enforce data integrity rules in a relational database.
ð Preview
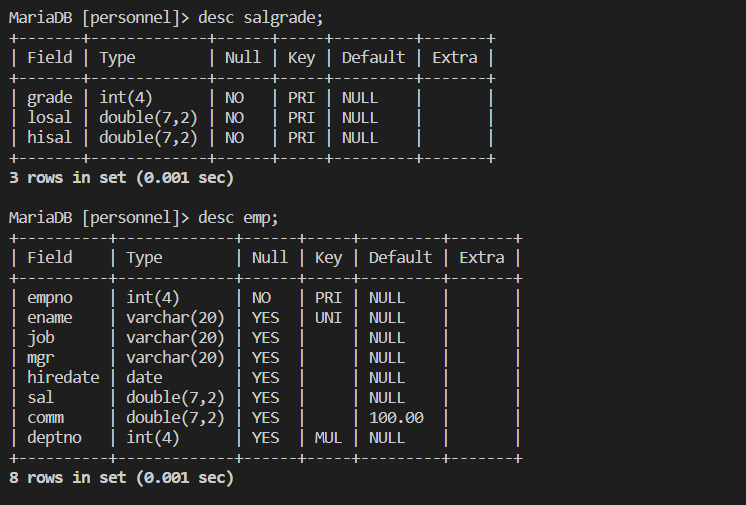
ðŊ Tasks
In this project, you will learn:
- How to create a composite primary key on the
salgrade
table - How to specify a foreign key on the
deptno
field in theemp
table - How to use a unique constraint to prevent duplicates in the
ename
field in theemp
table - How to set a default value for the
comm
field in theemp
table - How to use a check constraint to prevent the entry of hire dates later than February 28, 2022 in the
hiredate
field in theemp
table
ð Achievements
After completing this project, you will be able to:
- Understand the importance of data integrity constraints in database design
- Implement various types of constraints in a MySQL database
- Apply best practices for maintaining data quality and consistency
- Troubleshoot and debug issues related to database constraints