Introduction
In this project, you will learn how to build a shopping cart functionality using Vue.js 2.x. The shopping cart is an essential feature in e-commerce websites, allowing users to manage their selected products before making a purchase.
👀 Preview
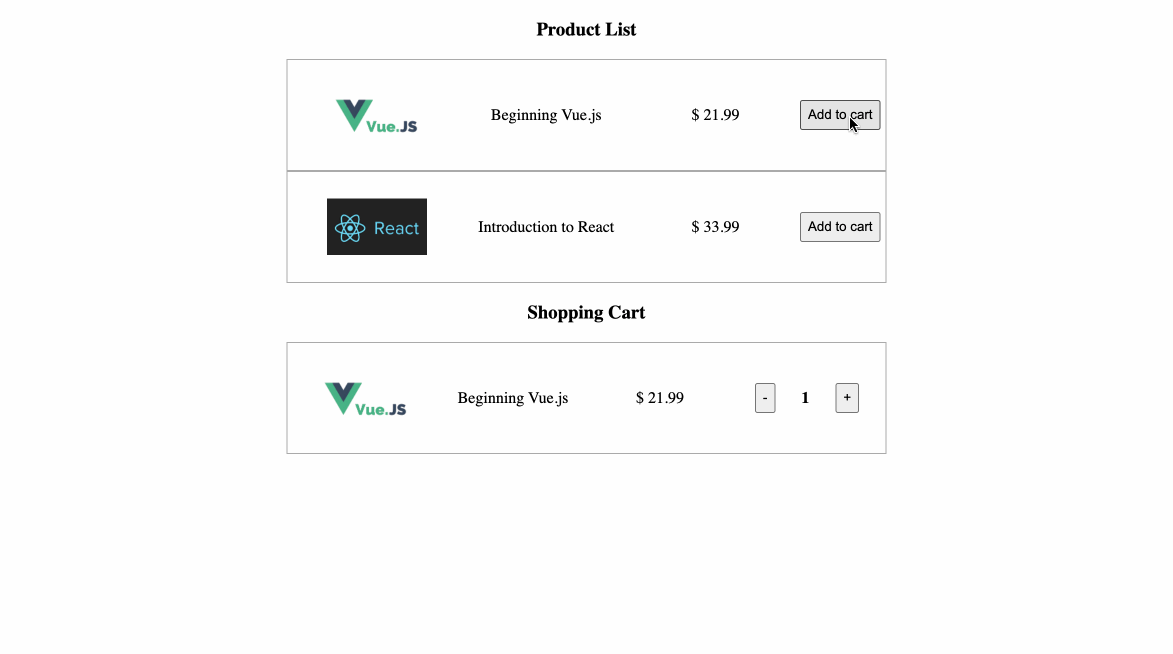
🎯 Tasks
In this project, you will learn:
- How to modify the
addToCart
method to add products to the shopping cart - How to improve the
removeGoods
method to remove products from the shopping cart - How to test the overall shopping cart functionality
🏆 Achievements
After completing this project, you will be able to:
- Manage the state of a shopping cart in a Vue.js application
- Handle the addition and removal of products in the shopping cart
- Update the user interface based on the changes in the shopping cart