Introduction
This project will guide you through the process of creating a VSCode extension that lets the user adjust the heading level of selected Markdown text. By the end, you'll have a functional extension that enhances the Markdown editing experience in Visual Studio Code.
👀 Preview
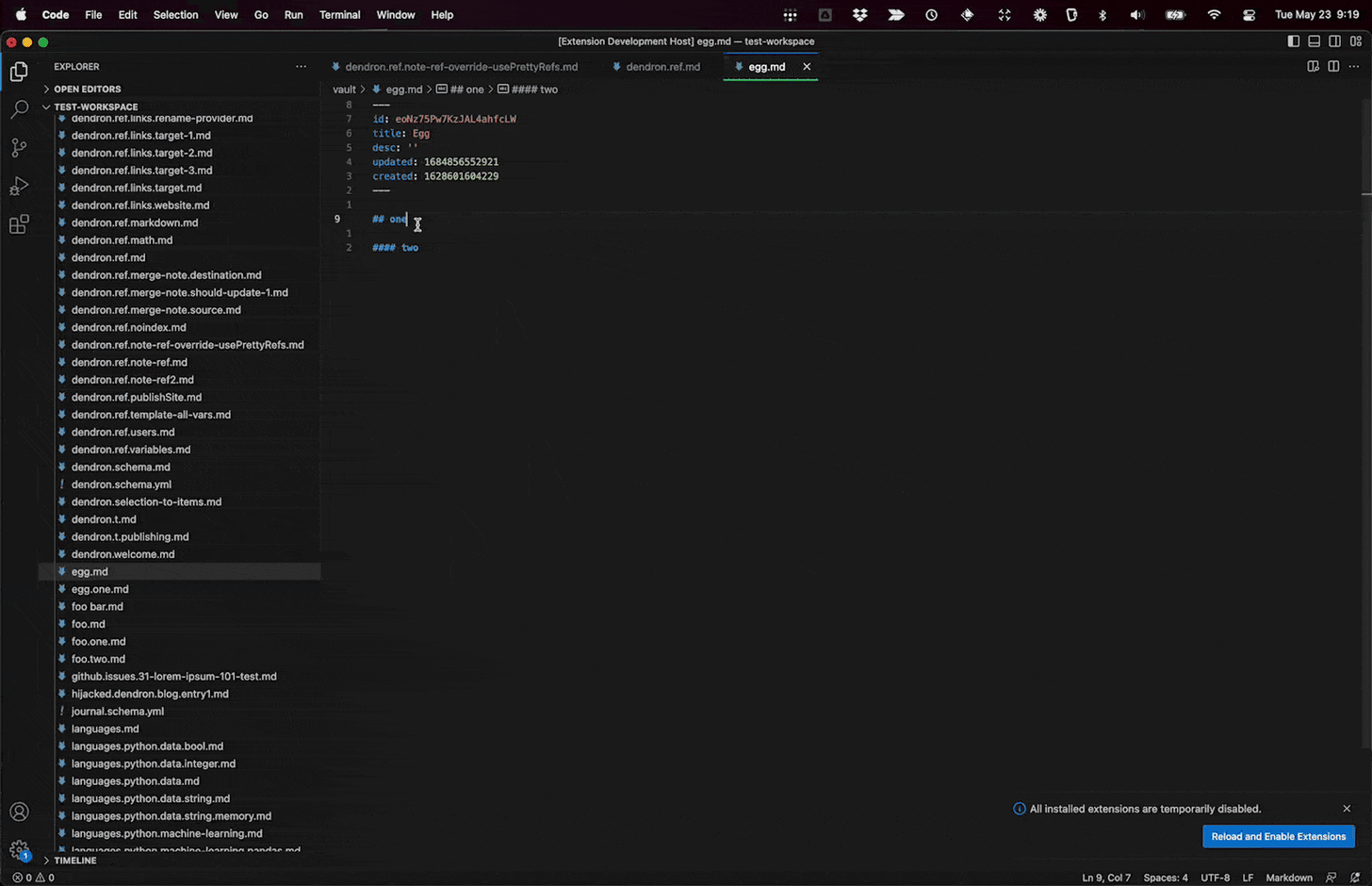
🎯 Tasks
In this project, you will learn:
- How to create the scaffolding for a VSCode extension using TypeScript.
- How to integrate TypeScript with Node.js and VSCode configurations.
- How to develop the core functionality to adjust heading levels of selected Markdown text.
- How to write configuration files for building, debugging, and running the extension.
🏆 Achievements
After completing this project, you will be able to:
- Design and develop VSCode extensions using TypeScript.
- Handle user interactions and text selections within the VSCode environment.
- Parse and manipulate Markdown syntax.
- Generate and manage multiple files in a project.
- Configure TypeScript, Node.js, and VSCode for seamless development.