Introduction
In this project, you will learn how to set up a Druid database connection pool and use it to retrieve data from a MySQL database. Druid is a popular open-source database connection pool that supports various database connections, including MySQL, PostgreSQL, Oracle, and more.
👀 Preview
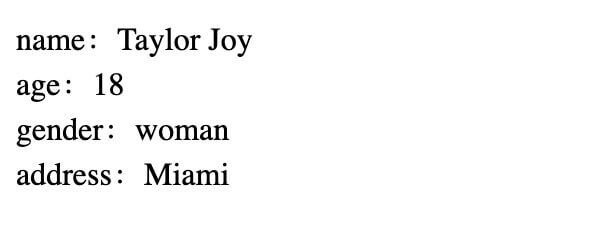
🎯 Tasks
In this project, you will learn:
- How to set up the Druid database connection pool
- How to implement the
getConn()
method to return a database connection from the Druid connection pool - How to retrieve data from the MySQL database using the Druid connection pool
🏆 Achievements
After completing this project, you will be able to:
- Configure and use the Druid database connection pool
- Interact with a MySQL database using a connection pool
- Apply best practices for managing database connections in a Java web application