Introduction
In this project, you will learn how to implement an employee information retrieval feature using the MVC architecture and Servlet2.x. You will create a search box on the index page where users can enter the employee ID to search, and then display the employee information on a separate page.
ð Preview
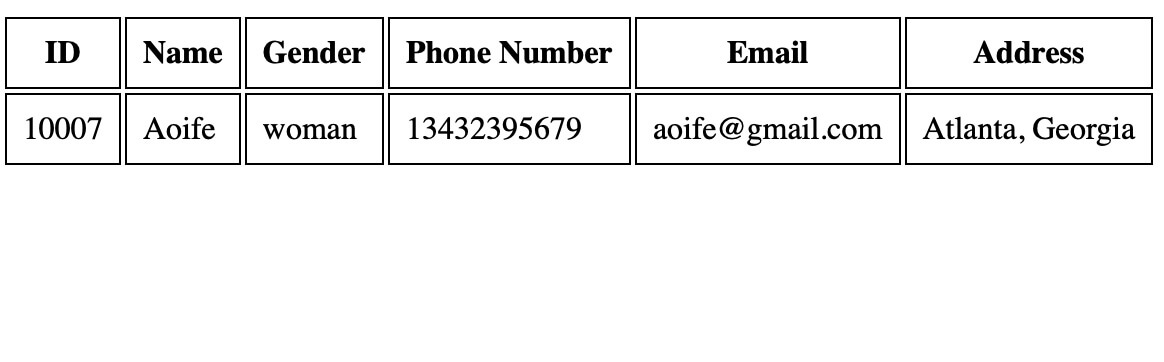
ðŊ Tasks
In this project, you will learn:
- How to create a search box on the index page to allow users to enter the employee ID
- How to implement the entity class to represent the employee data
- How to implement the JDBC utility class to get a database connection
- How to implement the DAO class to retrieve employee information from the database
- How to implement the controller class to handle the requests and forward the employee data to the JSP page
- How to implement the JSP page to display the queried employee information
ð Achievements
After completing this project, you will be able to:
- Use the MVC architecture to structure your web application
- Use Servlet2.x to handle HTTP requests and responses
- Interact with a database using JDBC
- Use JSP to display dynamic data on a web page