Introduction
In this project, you will learn how to configure a Spring Boot application using a YAML file, implement a class to handle the configuration data, and create a controller to display the configured information on a web page.
๐ Preview
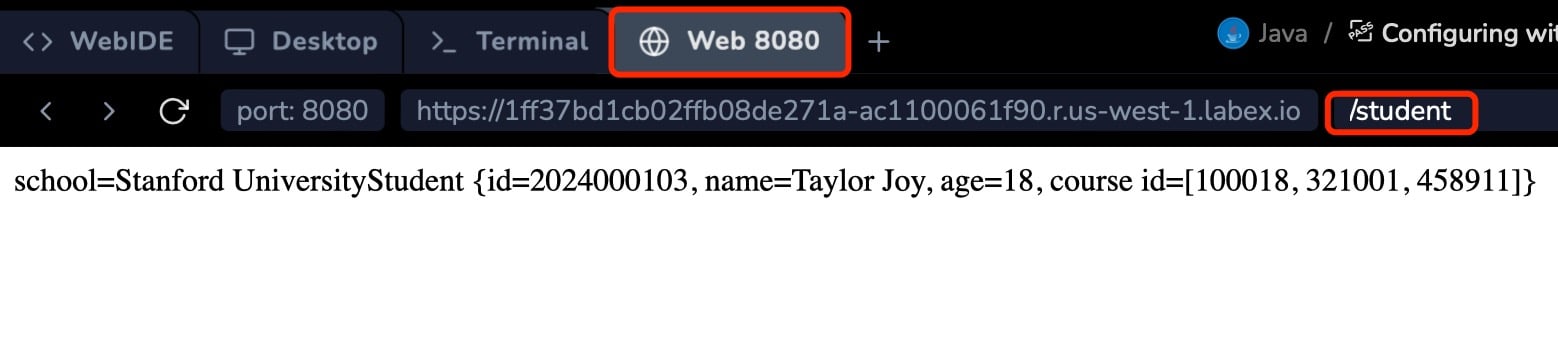
๐ฏ Tasks
In this project, you will learn:
- How to configure the application properties using a YAML file
- How to implement a
Student
class to handle the configuration data - How to create a
StudentController
to display the configured data on a web page - How to modify the startup class to scan all classes in the project package
๐ Achievements
After completing this project, you will be able to:
- Use YAML files for application configuration
- Use the
@ConfigurationProperties
annotation to automatically bind configuration data to a class - Use the
@Value
annotation to inject configuration values into a controller - Use the
@SpringBootApplication
annotation to configure component scanning - Develop a simple web application that displays the configured data