Introduction
2048 is a popular number puzzle game where the goal is to reach the 2048 tile by merging adjacent tiles with the same number. In this project, you'll learn how to create a simple 2048 game in C. We'll provide step-by-step instructions to build the game, from initializing the board to implementing game logic and running the game.
ð Preview
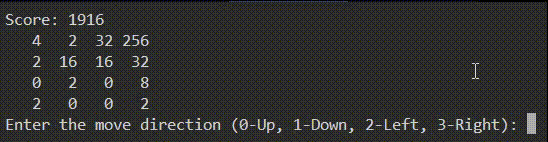
ðŊ Tasks
In this project, you will learn:
- How to create the project files
- How to define constants for the game
- How to implement the
main()
function to run the game loop - How to initialize the game board
- How to implement functions to check game state
- How to create the logic for moving tiles
- How to display the game board
- How to compile and test the game
ð Achievements
After completing this project, you will be able to:
- Create a C program for a game
- Use arrays to represent the game board
- Implement game logic for merging tiles
- Display the game board
- Handle player input
- Check for game over and victory conditions