Introduction
In this project, you will learn how to create a React application that allows users to switch between light and dark mode. The project will demonstrate the usage of the React Context API and the useContext
hook to manage the global theme state.
ð Preview
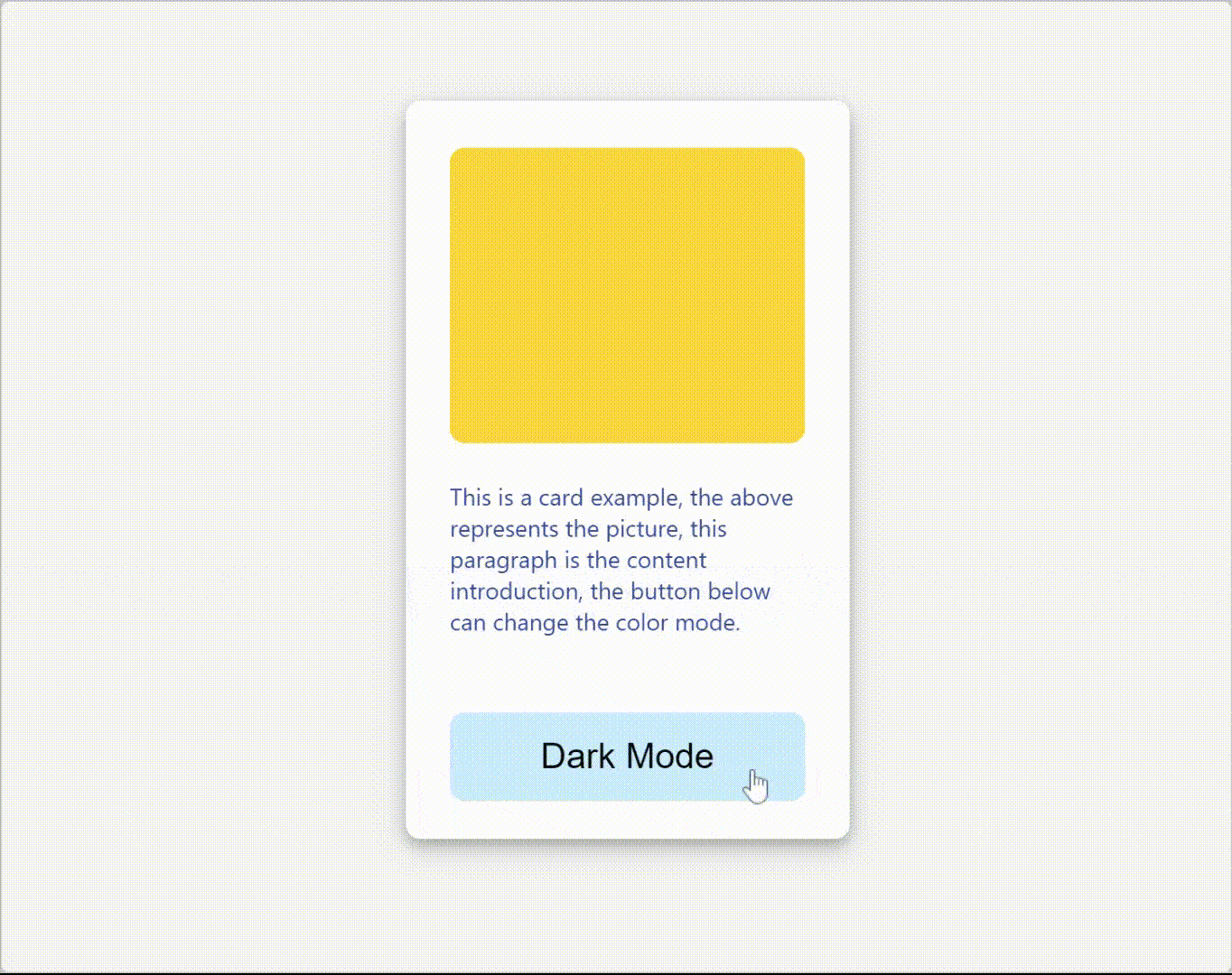
ðŊ Tasks
In this project, you will learn:
- How to create a
ThemeContext
using React's Context API - How to use the
ThemeContext
in theApp
component to change the overall app style based on the theme - How to use the
ThemeContext
in theCard
component to change the card styles based on the theme - How to wrap the
App
component with theThemeProvider
to make the theme context available throughout the application
ð Achievements
After completing this project, you will be able to:
- Understand how to use the React Context API to manage global state
- Apply the
useContext
hook to access the context values - Implement different styles based on the current theme
- Toggle the theme and update the global state