Introduction
In this project, you will learn how to build a simple IP visitor tracking application using Java Servlet and JSP. The application will keep track of the IP addresses of visitors accessing the website and the number of times each IP address has visited.
Preview
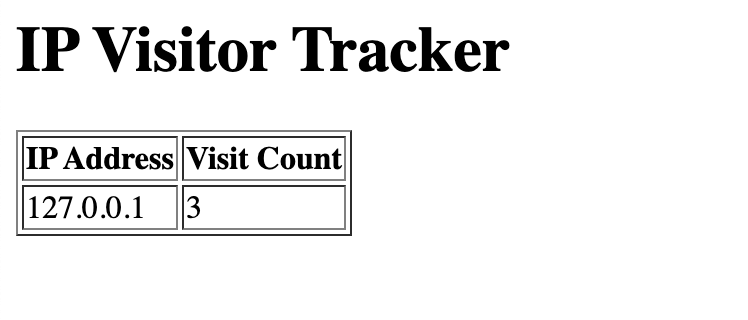
ðŊ Tasks
In this project, you will learn:
- How to implement the
index.jsp
file to display the IP addresses and their visit counts. - How to implement the
MyListener
class to create and store theipVisitMap
in theServletContext
. - How to implement the
MyFilter
class to count the number of visits for each IP address and update theipVisitMap
.
ð Achievements
After completing this project, you will be able to:
- Build a simple web application using Java Servlet and JSP.
- Use the
ServletContext
to store and retrieve application-level data. - Use a
Filter
to intercept and process incoming requests. - Use synchronization to ensure thread-safe access to shared resources.