Introduction
In this project, you will learn how to set up a Spring development environment using Maven and the Spring Framework version 5.3.7. This project will guide you through the process of creating a Maven project, configuring the Spring context, and writing a test class to verify the successful setup of the Spring environment.
ð Preview
- The directory structure should look like this:
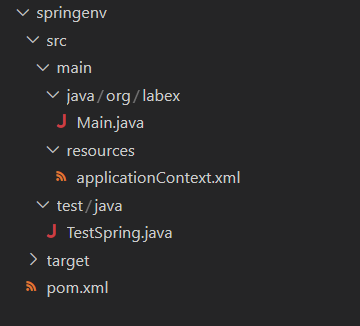
- The Spring environment was set up correctly:
[INFO] Scanning for projects...
[INFO]
[INFO] ------------------< org.labex:springenv >-------------------
[INFO] Building springenv 1.0-SNAPSHOT
[INFO] --------------------------------[ jar ]---------------------------------
[INFO]
[INFO] --- maven-resources-plugin:2.6:resources (default-resources) @ springenv ---
[INFO] Using 'UTF-8' encoding to copy filtered resources.
[INFO] Copying 1 resource
[INFO]
[INFO] --- maven-compiler-plugin:3.1:compile (default-compile) @ springenv ---
[INFO] Nothing to compile - all classes are up to date
[INFO]
[INFO] --- maven-surefire-plugin:2.12.4:test (default-test) @ springenv ---
[INFO] Surefire report directory: /home/labex/project/springenv/target/surefire-reports
-------------------------------------------------------
T E S T S
-------------------------------------------------------
Running org.labex.TestSpring
The Spring environment was built successfully!
Tests run: 1, Failures: 0, Errors: 0, Skipped: 0, Time elapsed: 0.662 sec
Results :
Tests run: 1, Failures: 0, Errors: 0, Skipped: 0
[INFO] ------------------------------------------------------------------------
[INFO] BUILD SUCCESS
[INFO] ------------------------------------------------------------------------
[INFO] Total time: 2.270 s
[INFO] Finished at: 2023-04-10T10:00:00Z
[INFO] ------------------------------------------------------------------------
ðŊ Tasks
In this project, you will learn:
- How to set up a Maven project for the Spring environment
- How to create the Spring configuration file
applicationContext.xml
- How to write a test class to validate the Spring environment setup
ð Achievements
After completing this project, you will be able to:
- Create a Maven project and manage dependencies using the
pom.xml
file - Configure the Spring context using the
applicationContext.xml
file - Write a test class to ensure the proper setup of the Spring environment