Introduction
This project will guide you through the process of creating a snake game using Python and the Pygame library. The game will have a game window, a snake, a power-up, and a score. The snake will move around the game window and eat the power-up. When the snake eats the power-up, the length of the snake will be increased by one. The score will be displayed on the screen.
👀 Preview
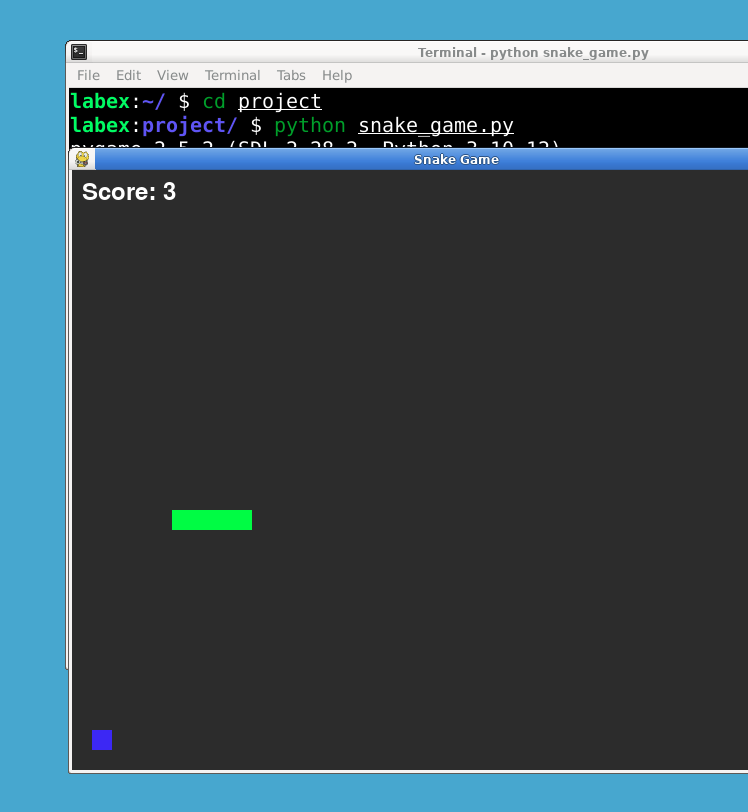
🎯 Tasks
In this project, you will learn:
- How to create a game window using Pygame
- How to handle user input to control the snake's movement
- How to create and update the snake's position
- How to draw the snake and the power-up on the screen
- How to detect collisions between the snake and the power-up
- How to keep track of the score
- How to display the score on the screen
🏆 Achievements
After completing this project, you will be able to:
- Use Pygame to create a graphical game window
- Handle user input to control the game
- Create and update game objects
- Detect collisions in a game
- Display and update the game score