Introduction
In this project, you will learn how to create an interactive jigsaw puzzle game using JavaScript. The game involves rearranging the puzzle pieces to reconstruct the complete image, providing an engaging and challenging experience for the players.
👀 Preview
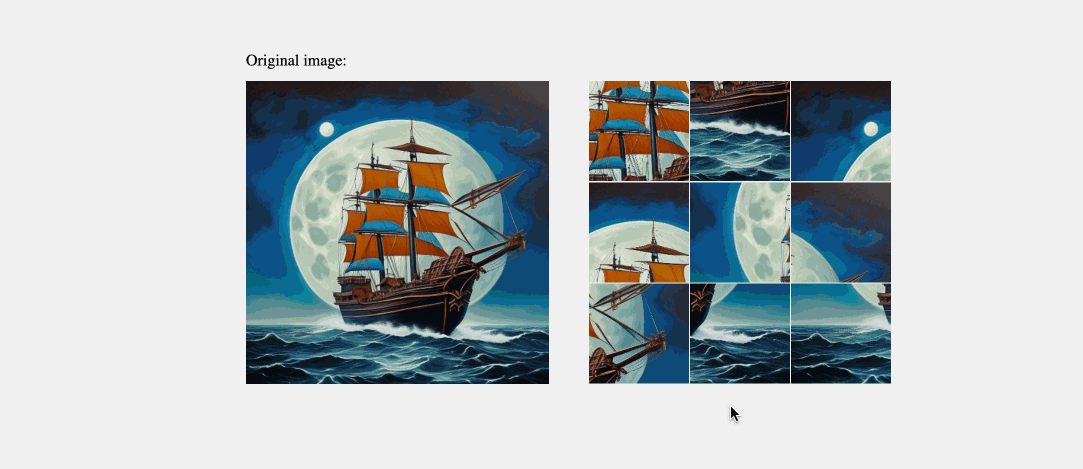
🎯 Tasks
In this project, you will learn:
- How to set up the project environment and understand the file structure.
- How to implement the drag and drop functionality for the puzzle pieces.
- How to check for the completion of the puzzle and display a success message accordingly.
- How to test the completed project and ensure the correct functionality of the game.
🏆 Achievements
After completing this project, you will be able to:
- Create an interactive game using JavaScript.
- Understand the principles of drag and drop functionality in web development.
- Implement logic to check for the completion of a task and provide feedback to the user.
- Gain experience in working with the Document Object Model (DOM) and manipulating HTML elements.