Introduction
In this project, you will learn how to implement a web page access tracking functionality using a listener. The goal of this project is to create a web application that can display the number of users currently accessing the home page.
👀 Preview
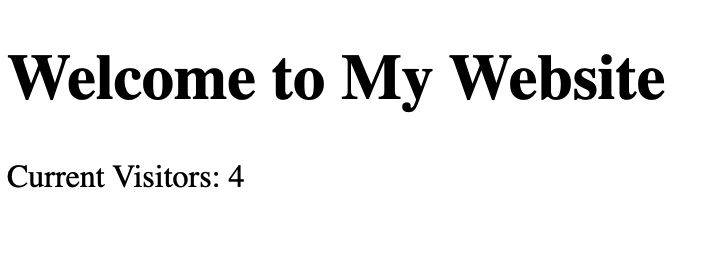
🎯 Tasks
In this project, you will learn:
- How to implement the
MyListener.java
class to track the number of hits to the home page. - How to implement the
index.jsp
file to display the current visitors count. - How to configure the
MyListener
in theweb.xml
file. - How to start the Tomcat server and test the application.
🏆 Achievements
After completing this project, you will be able to:
- Use the
HttpSessionListener
interface to track user sessions. - Store and retrieve the visitors count in the
ServletContext
. - Display the visitors count in a JSP file.
- Configure a listener in the
web.xml
file. - Start and test a web application using Tomcat.