Introduction
In this project, you will learn how to build a simple task manager application using Vue.js. The task manager allows you to create, delete, and keep track of your daily tasks, helping you become a time management master.
👀 Preview
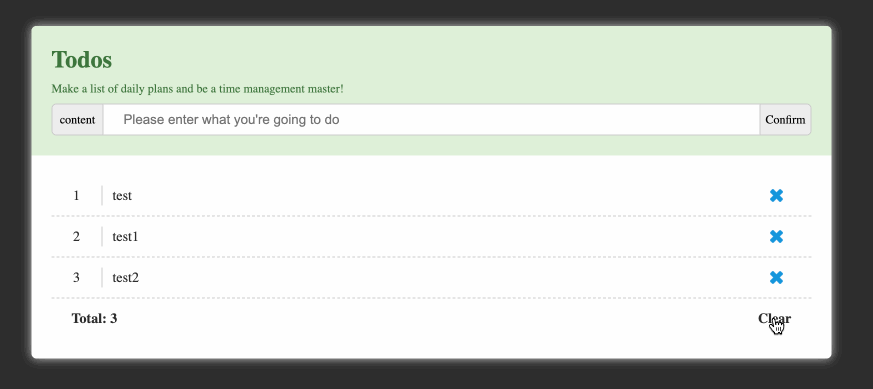
🎯 Tasks
In this project, you will learn:
- How to set up the Vue.js environment
- How to display "No data" by default when the page is loaded
- How to add tasks to the task list
- How to delete tasks from the task list
- How to display the total number of tasks
- How to clear the entire task list
🏆 Achievements
After completing this project, you will be able to:
- Create a Vue.js application from scratch
- Use Vue.js directives and data binding
- Implement basic CRUD (Create, Read, Update, Delete) functionality
- Handle user interactions and update the UI accordingly