Introduction
In this project, you will learn how to optimize database queries to improve performance in the LabEx system. The LabEx system is a platform that manages user study records, courses, and other related data. The project focuses on optimizing three frequently used queries in the system.
ð Preview
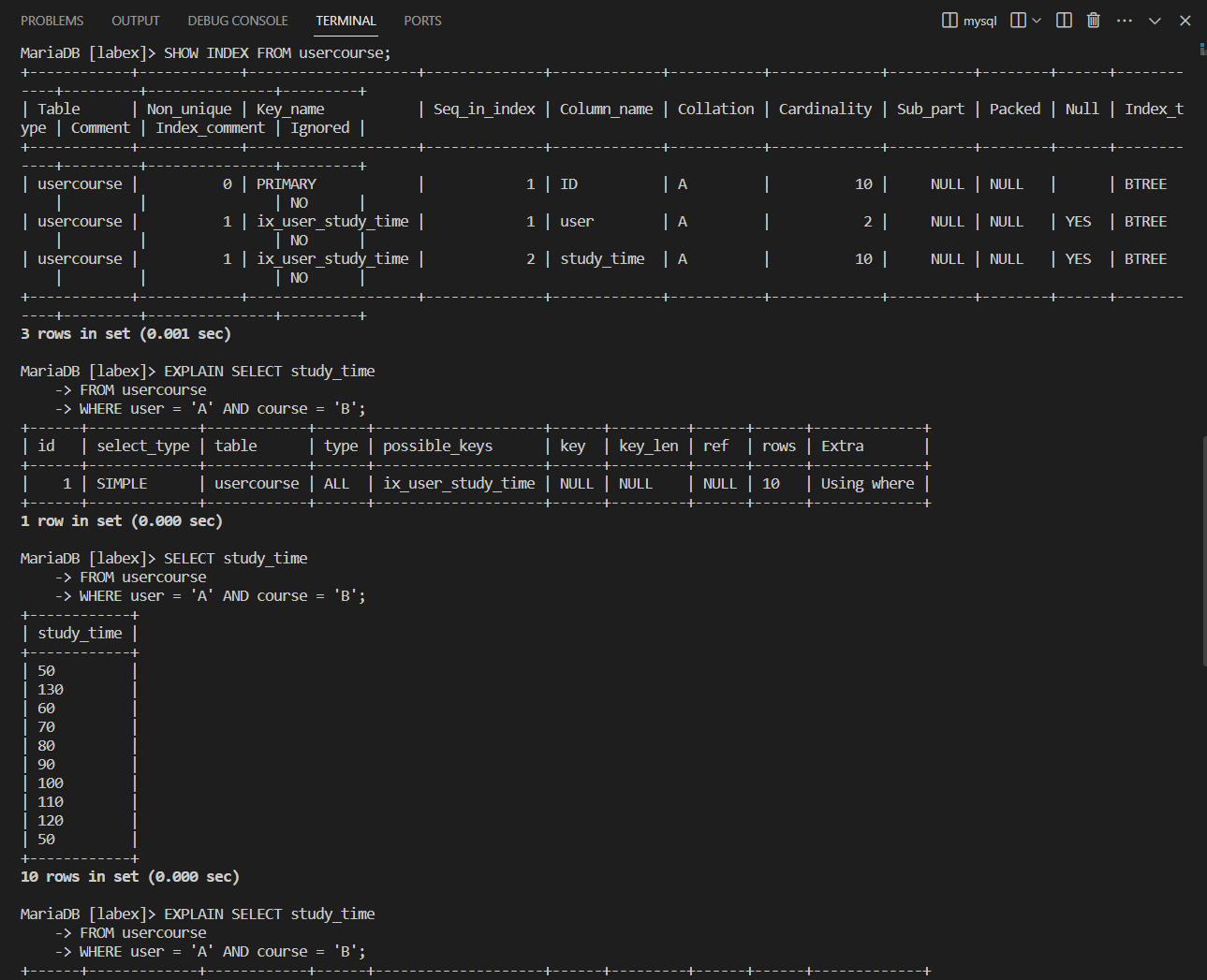
ðŊ Tasks
In this project, you will learn:
- How to add a compound index on the
user
andstudy_time
fields of theusercourse
table to improve query performance. - How to optimize the first query to find the study time of a specific user for a specific course.
- How to optimize the second query to sort the study records of a user's courses by study time.
- How to optimize the third query to retrieve all the courses that a user has studied.
ð Achievements
After completing this project, you will be able to:
- Understand the importance of indexing in database optimization.
- Implement compound indexes to improve the performance of complex queries.
- Analyze query execution plans to identify performance bottlenecks.
- Optimize database queries by leveraging appropriate indexes.
- Apply your knowledge to improve the performance of real-world database applications.