Introduction
In this project, you will create a Linux system monitor using a shell script. This script will continuously track the CPU, memory, and disk usage of your system, displaying the usage percentages in real time. If the usage of any resource exceeds a preset threshold, an alert will be displayed. By completing this project, you will learn foundational Linux scripting skills while building a practical tool.
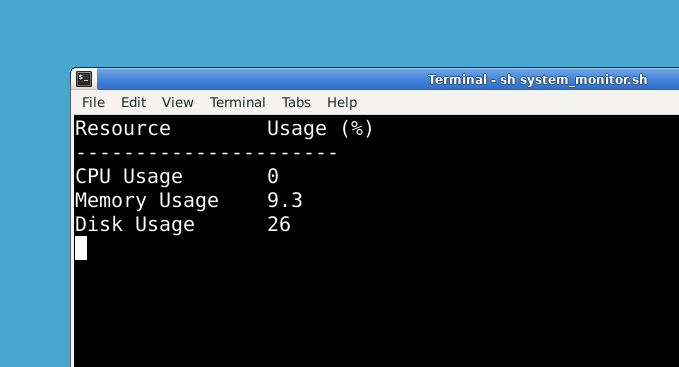
🎯 Tasks
By completing this project, you will:
- Learn how to create a shell script to monitor system resources.
- Understand how to set and use threshold values for CPU, memory, and disk usage.
- Create a function to send alerts when thresholds are exceeded.
🏆 Achievements
After completing this project, you will:
- Be able to create and run a Linux system monitor using a shell script.
- Understand how to work with system resource commands like
top
,free
, anddf
. - Be equipped to extend the script by adding new features, such as email notifications.